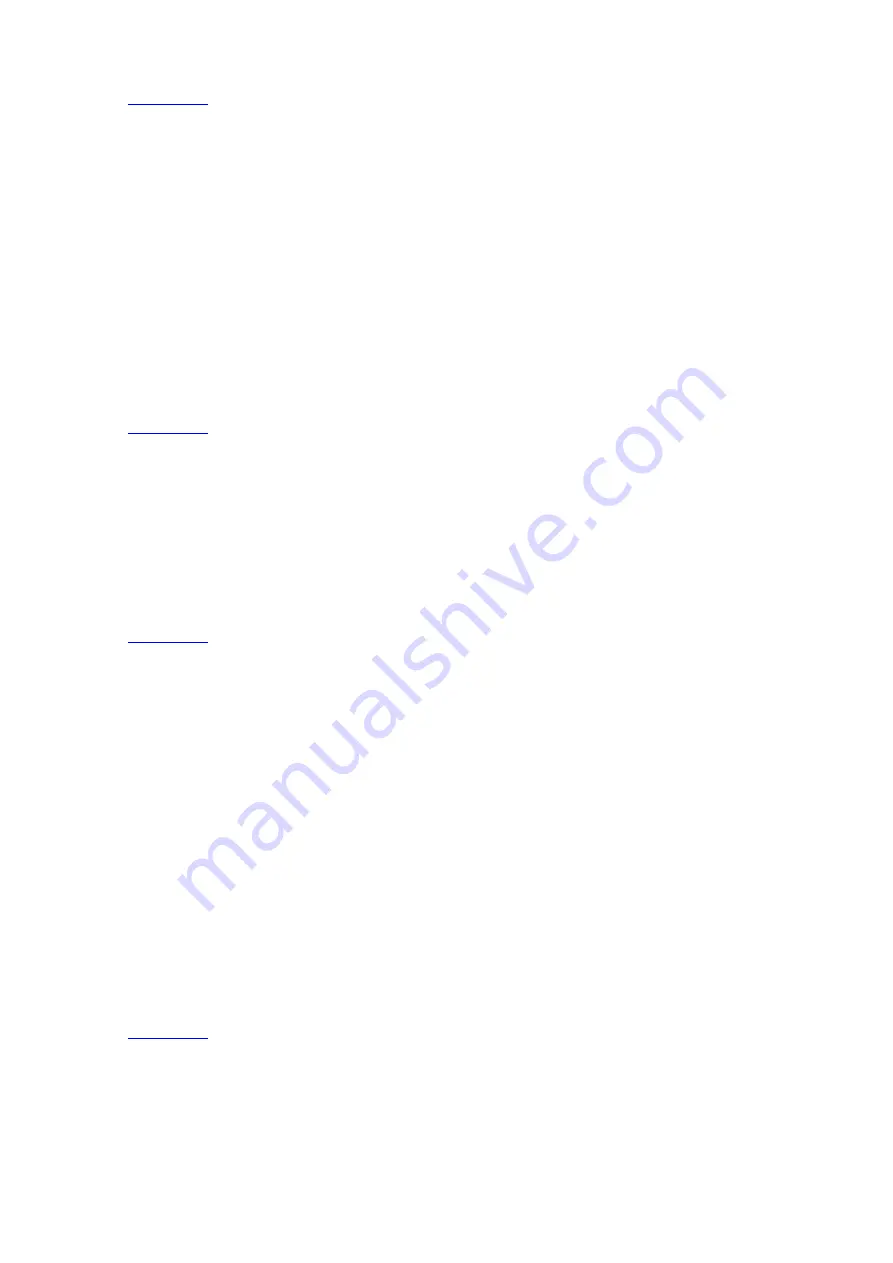
[Get Code]
Instantiate a server enabling the the Yun to listen for connected clients.
YunServer server;
In
setup()
, start serial communication for debugging purposes, and turn the built-in LED on pin
13 high while Bridge begins.
Bridge.begin()
is blocking, and should take about 2 seconds to
complete. Once Bridge starts up, turn the LED off.
void setup() {
Serial.begin(9600);
pinMode(13,OUTPUT);
digitalWrite(13, LOW);
Bridge.begin();
digitalWrite(13, HIGH);
[Get Code]
In the second part of
setup()
, tell the instance of YunServer to listen for incoming connections
only coming from localhost. Connections made to Linino will be passed to the 32U4 processor
for parsing and controlling the pins. This happens on port 5555. Start the server with
server.begin()
.
server.listenOnLocalhost();
server.begin();
}
[Get Code]
In
loop()
, you'll create an instance of the YunClient for managing the connection. If the client
connects, process the requests in a custom function (described below) and close the connection
when finished.
Putting a delay at the end of
loop()
will be helpful in keeping the processor from doing too
much work.
void loop() {
YunClient client = server.accept();
if (client) {
process(client);
client.stop();
}
delay(50);
}
[Get Code]
Create a function named
process
that accepts the YunClient as its argument. Read the command
by creating a string to hold the incoming information. Parse the REST commands by their
functionality (digital, analog, and mode) and pass the information to the appropriately named
function.