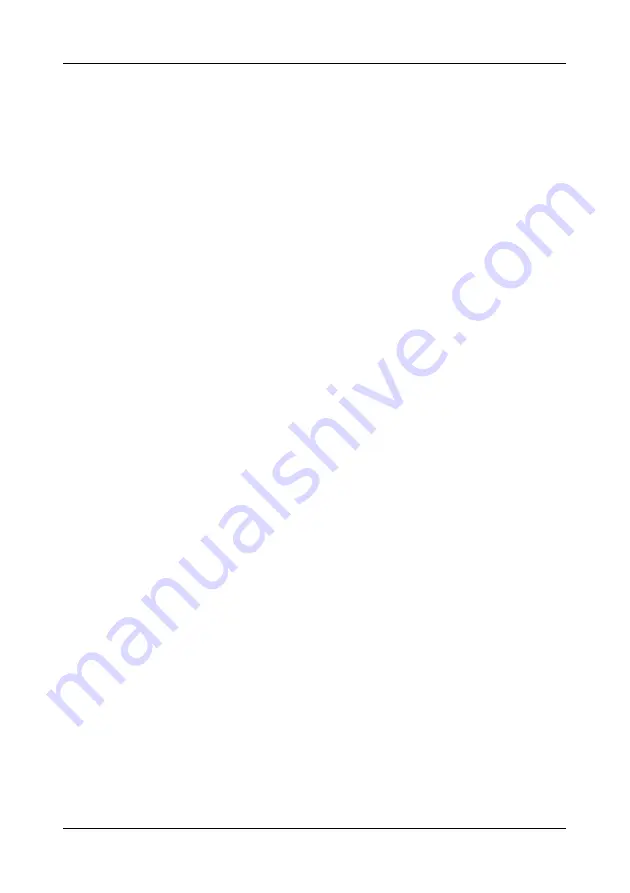
The following listing shows some basic input/output routines for
the RTC. They are based upon the SPI driver functions which are
shown above. For more complex functions, such as set date/time, please
refer to the software examples contained on the product CD.
For further instructions how to program the RTC, please refer to
the RTC4553 Application Manual.
//-----------------------------------------------------------------------------
// Func: getRTC()
// Args: regno[0..3] is RTC register number
// Retn: [0..3] RTC data (register contents)
// Note: there is no need to range check the regno argument since the
// RTC will ignore any additional bits anyway
//
UINT8 getRTC(UINT8 regno) {
UINT8 result;
// set up SPI mode: enable, master, CPOL=1, CPHA=1, LSB first
SPI0CR1 = BM_SPE | BM_MSTR | BM_CPOL | BM_CPHA | BM_LSBFE;
// transfer data
PTH = S12CO_SPICS0_RD; // enable /CS0 (reading)
xferSPI0(regno); // send register number to RTC
result = xferSPI0(0) >> 4; // receive data from RTC
PTH = S12CO_SPICSH; // disable all /CSx
return result;
}
//-----------------------------------------------------------------------------
// Func: putRTC()
// Args: regno[0..3] is RTC register number
// data[0..3] is RTC data (for that register)
// Retn: -
//
void putRTC(UINT8 regno, UINT8 data) {
// set up SPI mode: enable, master, CPOL=1, CPHA=1, LSB first
SPI0CR1 = BM_SPE | BM_MSTR | BM_CPOL | BM_CPHA | BM_LSBFE;
// transfer data
PTH = S12CO_SPICS0_WR; // enable /CS0 (writing)
xferSPI0((data << 4) | (regno & 0x0f));
PTH = S12CO_SPICSH; // disable all /CSx
}
//-----------------------------------------------------------------------------
On the S12compact, the RTC (including BT1) is an optional
component (not included in the standard version).
S12compact
28