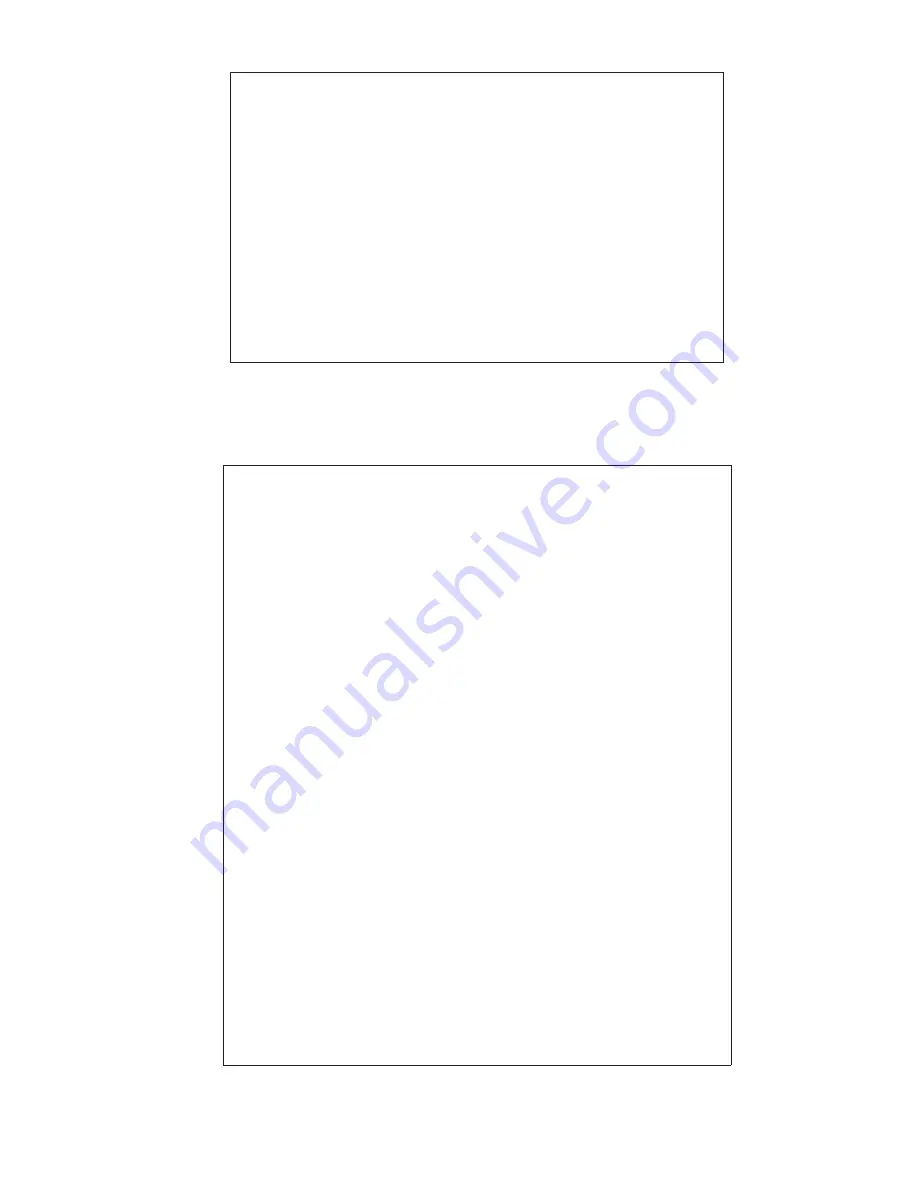
10
char LegacyChecksum(
char
* Response)
{
int length, sum =
0
, i, crc;
// Finding the length of the response string
length = strlen(Response);
// Adding characters in the response together
for
( i = 0; i < length; i++ ){
sum += Response[i];
}
// Converting checksum to a printable character
crc = sum %
64
+
32
;
return
crc;
}
The more robust
CRC6
, if available, utilizes the
CRC-6-CDMA2000-A
polynomial with the value
48
added to the
results to make this a printable character and is computed from the start of the transmission to the legacy
checksum character.
CRC6
checksum example input is
<TAB>
-34.8 22.3
<CR>
k@
and the resulting checksum output is
l
(lowercase L).
uint8_t
CRC6_Offset(
uint8_t
*buffer,
uint16_t
bytes)
{
uint16_t
byte;
uint8_t
bit;
uint8_t
crc =
0xfc
;
// Set upper 6 bits to 1’s
// Loop through all the bytes in the buffer
for
(byte =
0
; byte < bytes; byte++)
{
// Get the next byte in the buffer and XOR it with the crc
crc ^= buffer[byte];
// Loop through all the bits in the current byte
for
(bit =
8
; bit >
0
; bit--)
{
// If the uppermost bit is a 1...
if
(crc &
0x80
)
{
// Shift to the next bit and XOR it with a polynomial
crc = (crc <<
1
) ^
0x9c
;
}
else
{
// Shift to the next bit
crc = crc <<
1
;
}
}
}
// Shift upper 6 bits down for crc
crc = (crc >>
2
);
// Add 48 to shift crc to printable character avoiding \r \n and !
return
(crc +
48
);
}