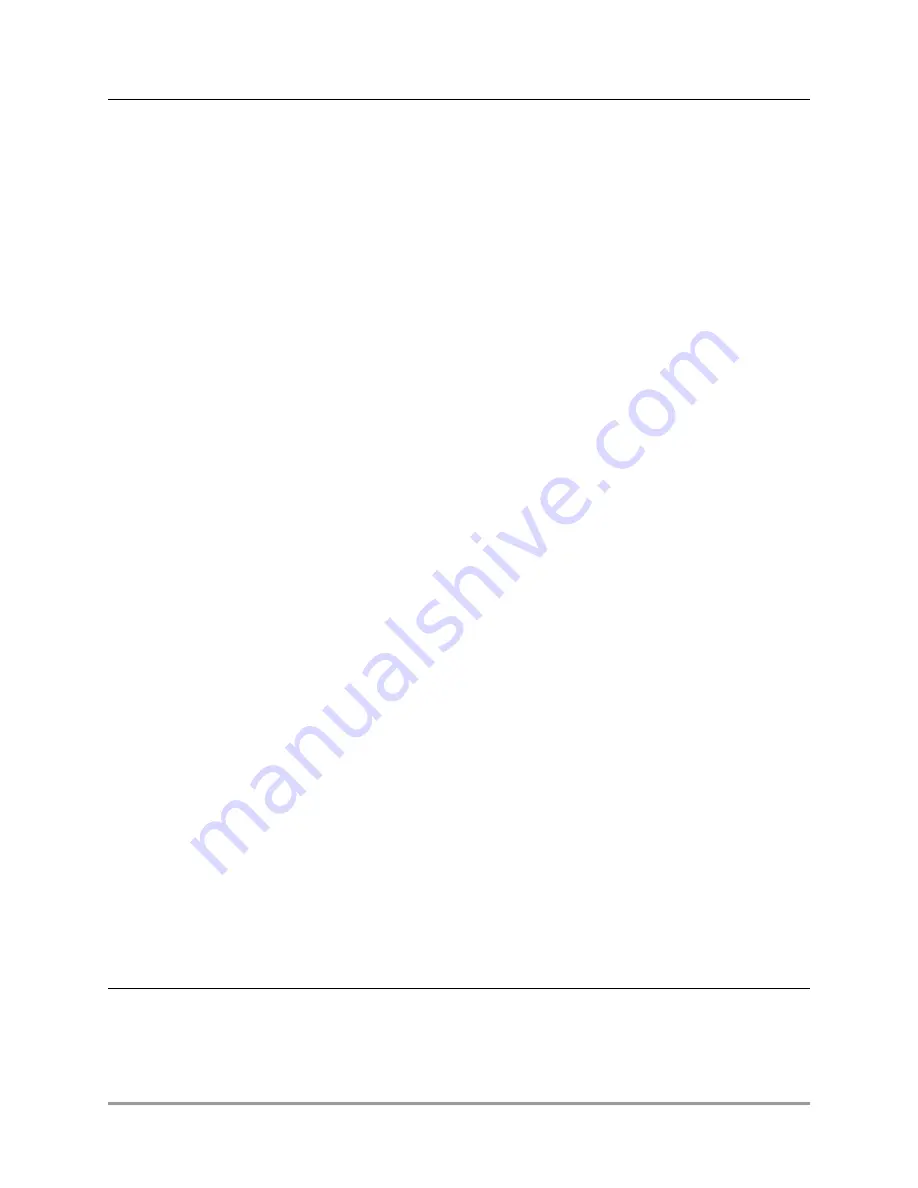
Complete Example of Profiling
A-3
Code A-3. EOnCE_stopwatch.c
/*
* Header file contains definitions of EOnCE memory-mapped register addresses,
* and definition of the WRITE_IOREG() macro.
*/
#include "EOnCE_registers.h"
#include "EOnCE_stopwatch.h"
unsigned long CLOCK_SPEED = 300000000;
static volatile long EOnCE_stopwatch_timer_flag; /*Global dummby variable*/
void EOnCE_stopwatch_timer_init()
{
WRITE_IOREG(EDCA1_REFA,(long)&EOnCE_stopwatch_timer_flag);
/* Address to snoop for on XABA */
WRITE_IOREG(EDCA1_REFB,(long)&EOnCE_stopwatch_timer_flag);
/* Address to snoop for on XABB */
WRITE_IOREG(EDCA1_MASK,MAX_32_BIT);
/* No masking is performed in address comparison */
WRITE_IOREG(EDCA1_CTRL,0x3f06);
/* Detect writes on both XABA and XABB */
}
void EOnCE_LED_init()
{
*((long *)EE_CTRL) &= ~(3<<2); /* Toggle EE1 when event1 happens */
}
void EOnCE_stopwatch_timer_start()
{
WRITE_IOREG(ECNT_VAL,MAX_32_BIT); /* Countdown will start at (2**32)-1 */
WRITE_IOREG(ECNT_EXT,0); /* Extension will count up from zero */
WRITE_IOREG(ECNT_CTRL,0x12c);
/* Counting will be triggered by detection on EDCA1 */
EOnCE_stopwatch_timer_flag = 0;
/* This write to the flag triggers the counter */
}
void EOnCE_stopwatch_timer_stop(unsigned long *clock_ext, unsigned long *clock_val)
{
WRITE_IOREG(ECNT_CTRL,0);
/* Disable event counter */
READ_IOREG(ECNT_VAL,*clock_val); /* Save ECNT_VAL in program variable */
READ_IOREG(ECNT_EXT,*clock_ext); /* Save ECNT_EXT in program variable */
*clock_val = (MAX_32_BIT-*clock_val); /* Adjust for countdown */
}
void EOnCE_LED_off()
{
EOnCE_stopwatch_timer_flag = 0; /* Create an EDCA1 event */
}
unsigned long Convert_clock2time(unsigned long clock_ext, unsigned long clock_val, short option)
{
unsigned long result;
switch(option)
{
case EONCE_SECOND:
result= clock_ext*MAX_32_BIT/CLOCK clock_val/CLOCK_SPEED;
break;
case EONCE_MILLISECOND:
result= clock_ext*MAX_32_BIT/(CLOCK_SPEED/1000)
+ clock_val/(CLOCK_SPEED/1000);
break;
case EONCE_MICROSECOND:
result= clock_ext*MAX_32_BIT/(CLOCK_SPEED/1000000)
+ clock_val/(CLOCK_SPEED/1000000);
break;
default: result=0; /* error condition */
break;
}
return result;
}