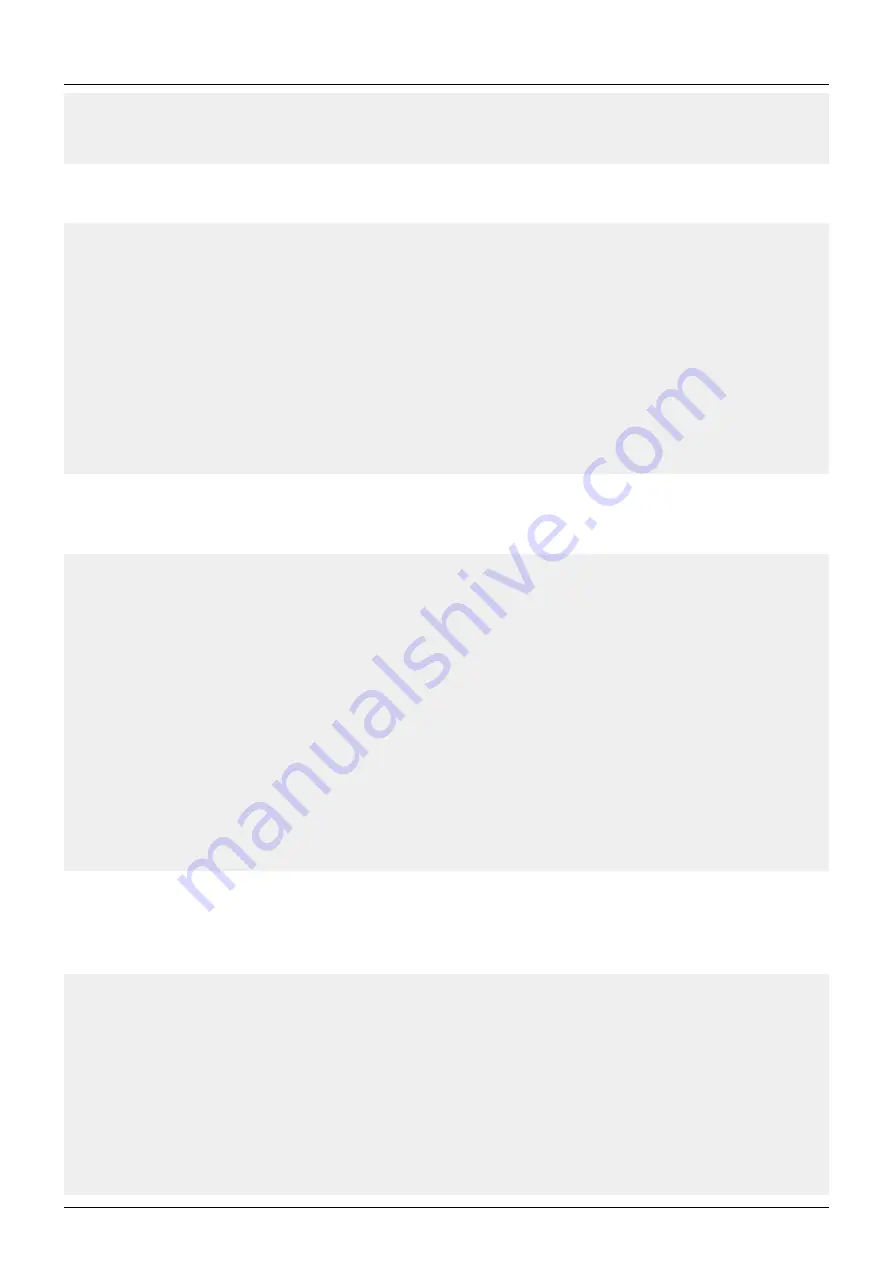
Last update:
2018/06/11
12:25
odroid_go:arduino:03_blue_led_and_pwm https://wiki.odroid.com/odroid_go/arduino/03_blue_led_and_pwm?rev=1528687521
https://wiki.odroid.com/
Printed on 2023/05/05 21:45
void
loop
()
{
// put your main code here, to run repeatedly:
}
And control the pin mode of the LED to output. It might be not a necessary step, but to make sure.
#define PIN_BLUE_LED 2
#define PWM_CHANNEL 1
void
setup
()
{
// put your setup code here, to run once:
pinMode
(
PIN_BLUE_LED
,
OUTPUT
);
}
void
loop
()
{
// put your main code here, to run repeatedly:
}
Attach the LED pin to the channel we defined.
Next, setup that channel runs in 12kHz, 8 bit resolution.
#define PIN_BLUE_LED 2
#define PWM_CHANNEL 1
void
setup
()
{
// put your setup code here, to run once:
pinMode
(
PIN_BLUE_LED
,
OUTPUT
);
ledcAttachPin
(
PIN_BLUE_LED
,
PWM_CHANNEL
);
ledcSetup
(
PWM_CHANNEL
,
12000
,
8
);
}
void
loop
()
{
// put your main code here, to run repeatedly:
}
Lastly, add a breathing code into the loop() function.
We defined a variable calls pinVal as a global variable to prevent from allocating a new memory
repeatedly in the loop() function.
#define PIN_BLUE_LED 2
#define PWM_CHANNEL 1
int
pinVal
=
0
;
void
setup
()
{
// put your setup code here, to run once:
pinMode
(
PIN_BLUE_LED
,
OUTPUT
);