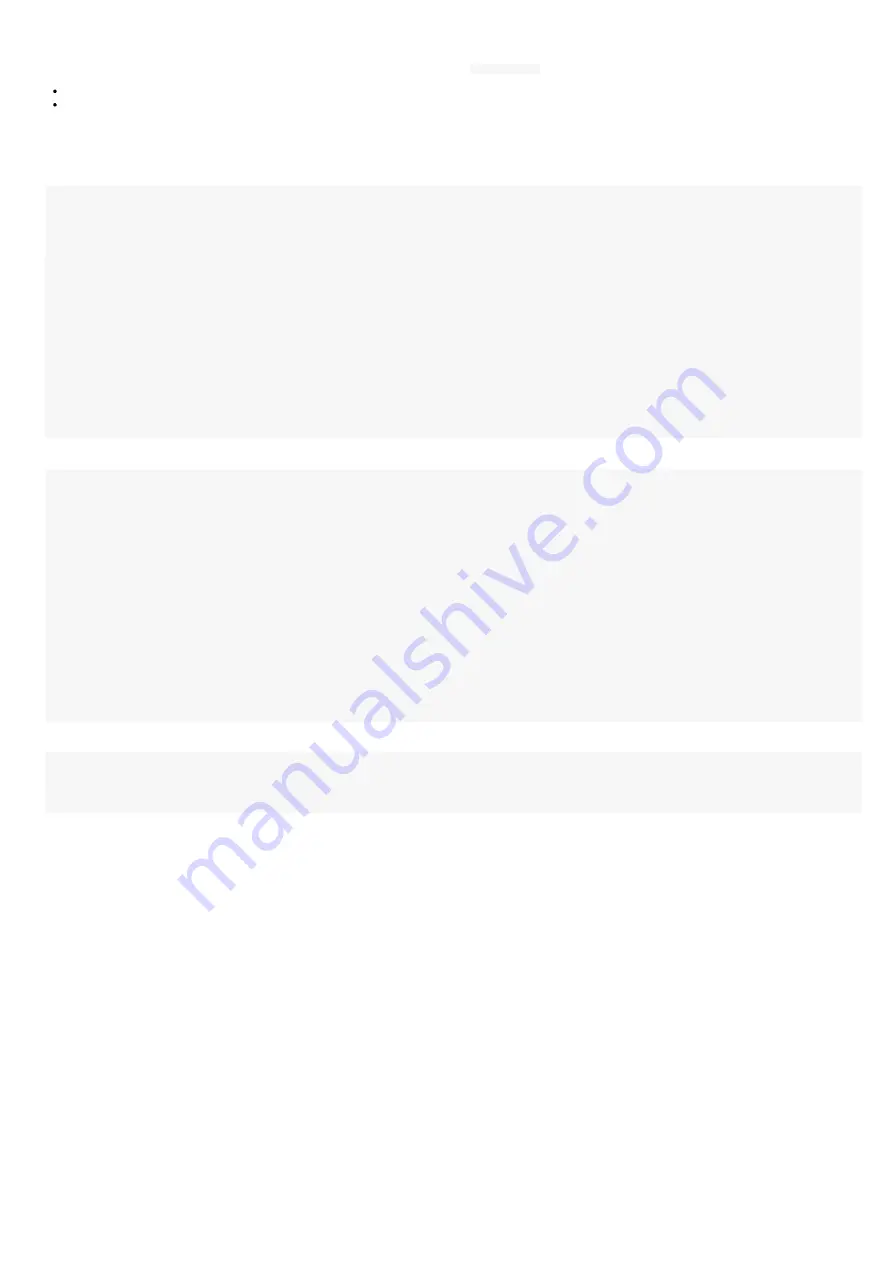
6
APK shared preferences handling with configura on script
An APK can be designed to share some preferences which can be then read or wri en by the
Qeedji System
service:
either through the configura on script,
or through the device configura on Web interface¹.
¹
Should be available Q2 /2 0 2 1 .
The APK must link the
tech-qeedji-system-lib-classes.jar
Java library.
The APK must also implement a child class of the
SharedPreferenceAPI
class supported in the
tech-qeedji-system-lib-classes.jar
Java library.
package tech.qeedji.test1;
import tech.qeedji.system.lib.SharedPreferenceAPI;
public class MySharedPreferenceAPI extends SharedPreferenceAPI {
@Override
public String getPreferenceAuthority() {
return BuildConfig.APPLICATION_ID;
}
@Override
public Object[][] initPreferences() {
Object[][] preferences = {
// filename, key, access, type, default_value
{"test1", "test1Integer", "rw", int.class, 1},
{"test1", "test1Long", "rw", long.class, 10000000L},
{"test1", "test1Float", "rw", float.class, 0.897546F},
{"test1", "test1Boolean", "rw", boolean.class, true},
{"test1", "test1String", "rw", String.class, "test1StringValue"},
{"test1", "test1StringSet", "rw", String[].class, new String[]{"http://Val0", "http://Val1", "http://Val2"}},
};
return preferences;
}
}
The APK must declare a provider in its manifest.
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="tech.qeedji.test1">
<application
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<provider android:name="tech.qeedji.test1.MySharedPreferenceAPI"
android:authorities="tech.qeedji.test1"
android:multiprocess="false"
android:exported="true"
android:singleUser="false"
android:initOrder="100"
android:visibleToInstantApps="true"/>
</application>
</manifest>
Extract example from a configura on script:
Android.Preferences("SharedPreferences", "tech.qeedji.test1", "test1").setInt("test1Integer", 8);
Android.Preferences("SharedPreferences", "tech.qeedji.test1", "test1").setLong("test1Long", 99999999);
Android.Preferences("SharedPreferences", "tech.qeedji.test1", "test1").setFloat("test1Float", 0.123456);
Android.Preferences("SharedPreferences", "tech.qeedji.test1", "test1").setBoolean("test1Boolean", false);
Android.Preferences("SharedPreferences", "tech.qeedji.test1", "test1").setString("test1String", "Newtest1StringValue");
Android.Preferences("SharedPreferences", "tech.qeedji.test1", "test1").setStringArray("test1StringSet", ["http://NewVal0", "http://NewVal1", "http://NewVal2"]);