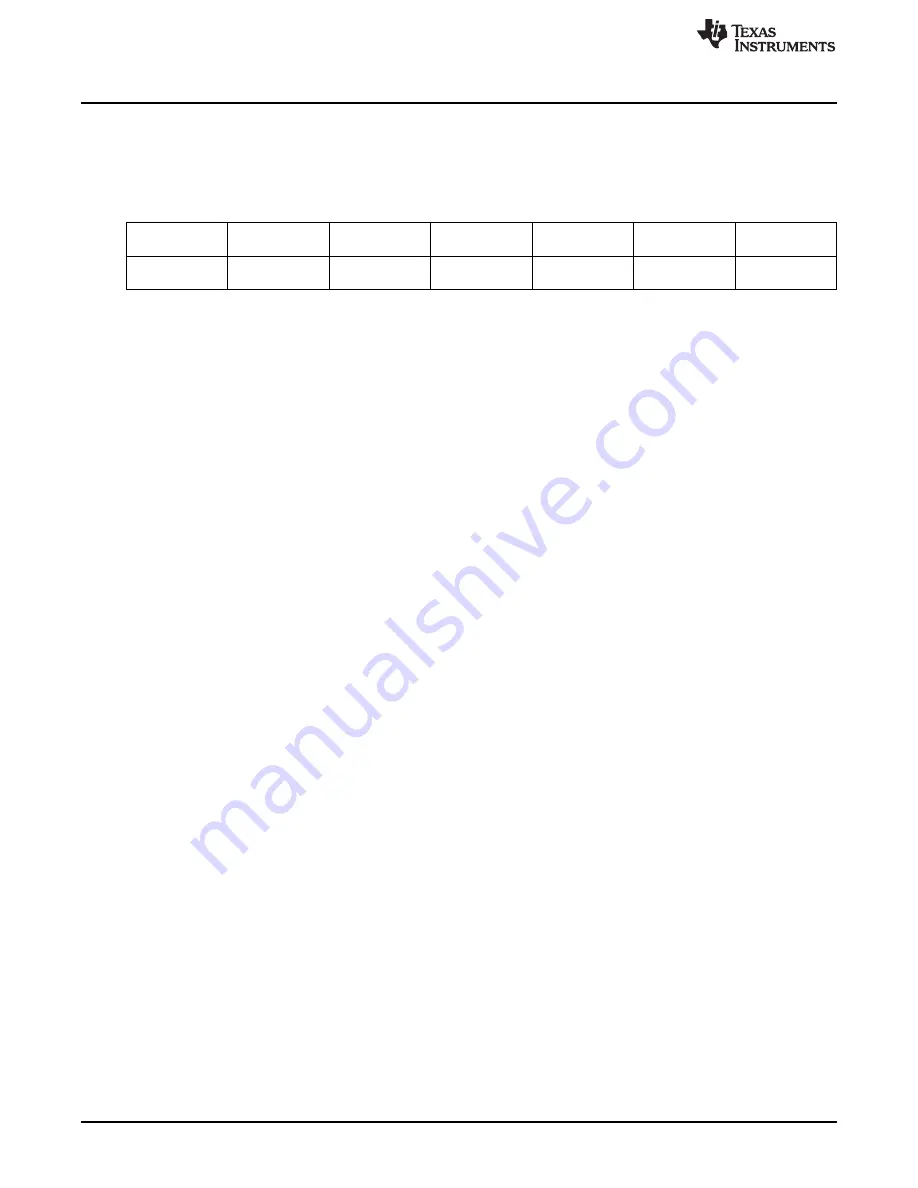
Host HTTP Requests Processing
156
SWRU455A – February 2017 – Revised March 2017
Copyright © 2017, Texas Instruments Incorporated
HTTP Server
The TLVs are packed continuously in the metadata section of the request. The user’s code should begin
parsing from byte 0, which is always the type field of the first TLV, and finish when metadata-length bytes
are processed (which should point to the last byte of the value field of the last TLV). The TLVs are packed
in no particular order.
is an example metadata breakout containing two TLVs
Table 9-25. Metadata Breakout Examples
Metadata Offset
/ Content
0 (TLV1 Type)
1–2 (TLV1
Length)
3–10 (TLV1
Value)
11 (TLV2 Type)
12–13 (TLV2
Length)
14–24 (TLV2
Value)
Data
1 (HTTP
Version)
11
“HTTP/1.0”
19 (Header Host)
14
10.123.45.1
An example of how to find and extract the content of a specific TLV from the metadata buffer follows:
_i32 ExtractLengthFromMetaData(_u8 *pMetaDataStart, _u16 MetaDataLen)
{
_u8 *pTlv;
_u8 *pEnd;
_u8 Type;
_u16 TlvLen;
pTlv = pMetaDataStart;
pEnd = pMetaDat MetaDataLen;
while
(pTlv < pEnd)
{
Type = *pTlv;
/* Type is one byte */
pTlv++;
TlvLen = *(_u16 *)pTlv;
/* Length is two bytes */
pTlv+=2;
if
(Type == SL_NETAPP_REQUEST_METADATA_TYPE_HTTP_CONTENT_LEN)
{
_i32 LengthFieldValue=0;
/* Found the right type, extract its value and return. */
memcpy(&LengthFieldValue, pTlv, TlvLen);
return LengthFieldValue;
}
else
{
/* Not the type we are looking for. Skip over the
value field to the next
type. */
pTlv += TlvLen;
}
}
return
-1;
}
/* NetApp request handler*/
void
NetAppRequestHandler(
SlNetAppRequest_t *pNetAppRequest,
SlNetAppResponse_t *pNetAppResponse)
{
_u32 HttpContentLength;
if
(pNetAppRequest->requestData.MetadataLen > 0)
{
HttpContentLength = ExtractLengthFromMetaData(
pNetAppRequest->requestData.pMetadata,
pNetAppRequest->requestData.MetadataLen);
}
}