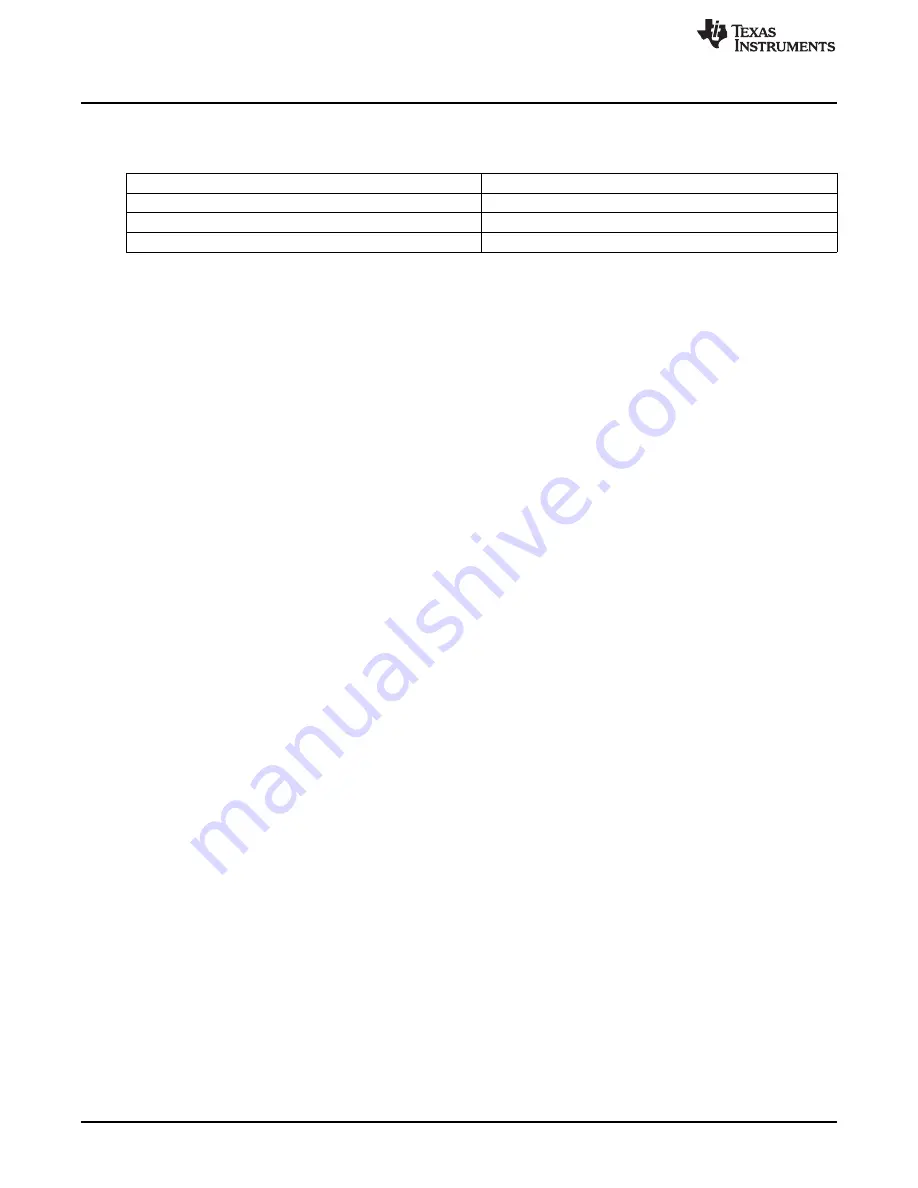
Socket Working Flow
88
SWRU455A – February 2017 – Revised March 2017
Copyright © 2017, Texas Instruments Incorporated
Socket
To join or leave a group, use sl_SetSockOpt with the options listed in
Table 6-3. Multicast
SL_IP_ADD_MEMBERSHIP
Join IPv4 group
SL_IP_DROP_MEMBERSHIP
Leave IPv4 group
SL_IPV6_ADD_MEMBERSHIP
Join IPv6 group
SL_IPV6_DROP_MEMBERSHIP
Leave IPv6 group
An example of joining the IPv4 multicast group:
_i16 Status;
SlSockIpMreq_t MulticastIp;
MulticastIp.imr_multiaddr.s_addr = sl_Htonl(SL_IPV4_VAL(224,0,1,200));
MulticastIp.imr_interface.s_addr = SL_INADDR_ANY;
Status = sl_SetSockOpt(Sd, SL_IPPROTO_IP, SL_IP_ADD_MEMBERSHIP,(
char
*) &MulticastIp,
sizeof
(MulticastIp));
if
( Status )
{
// error
}
An example of leaving the IPv4 multicast group:
_i16 Status;
SlSockIpMreq_t MulticastIp;
MulticastIp.imr_multiaddr.s_addr = sl_Htonl(SL_IPV4_VAL(224,0,1,200));
MulticastIp.imr_interface.s_addr = SL_INADDR_ANY;
Status = sl_SetSockOpt(Sd, SL_IPPROTO_IP, SL_IP_DROP_MEMBERSHIP,(
char
*) &MulticastIp,
sizeof
(MulticastIp));
if
( Status )
{
// error
}
6.5.2.2
Packet Boundary
By default the Rx boundary is kept. When the host application reads only a part of the data, the rest is
dropped. The host application can disable the Rx boundary by using sl_SetSockOpt with
SL_SO_RX_NO_IP_BOUNDARY. Here reading only a part of the data does not drop the rest of the data.
This is a propriety option for UDP sockets only, which enables the host with limited buffering resources to
read data in small chunks.
_i16 Status;
SlSockRxNoIpBoundary_t enableOption;
enableOption.RxIpNoBoundaryEnabled = 1;
Status = sl_SetSockOpt(Sd,SL_SOL_SOCKET,SL_SO_RX_NO_IP_BOUNDARY, (_u8*)&enableOption
,
sizeof
(enableOption));
if( Status )
{
// error
}
6.5.3 RAW
RAW sockets provide access to the underlying communication protocols with socket abstractions. The
working flow is very similar to a connectionless socket (UDP).