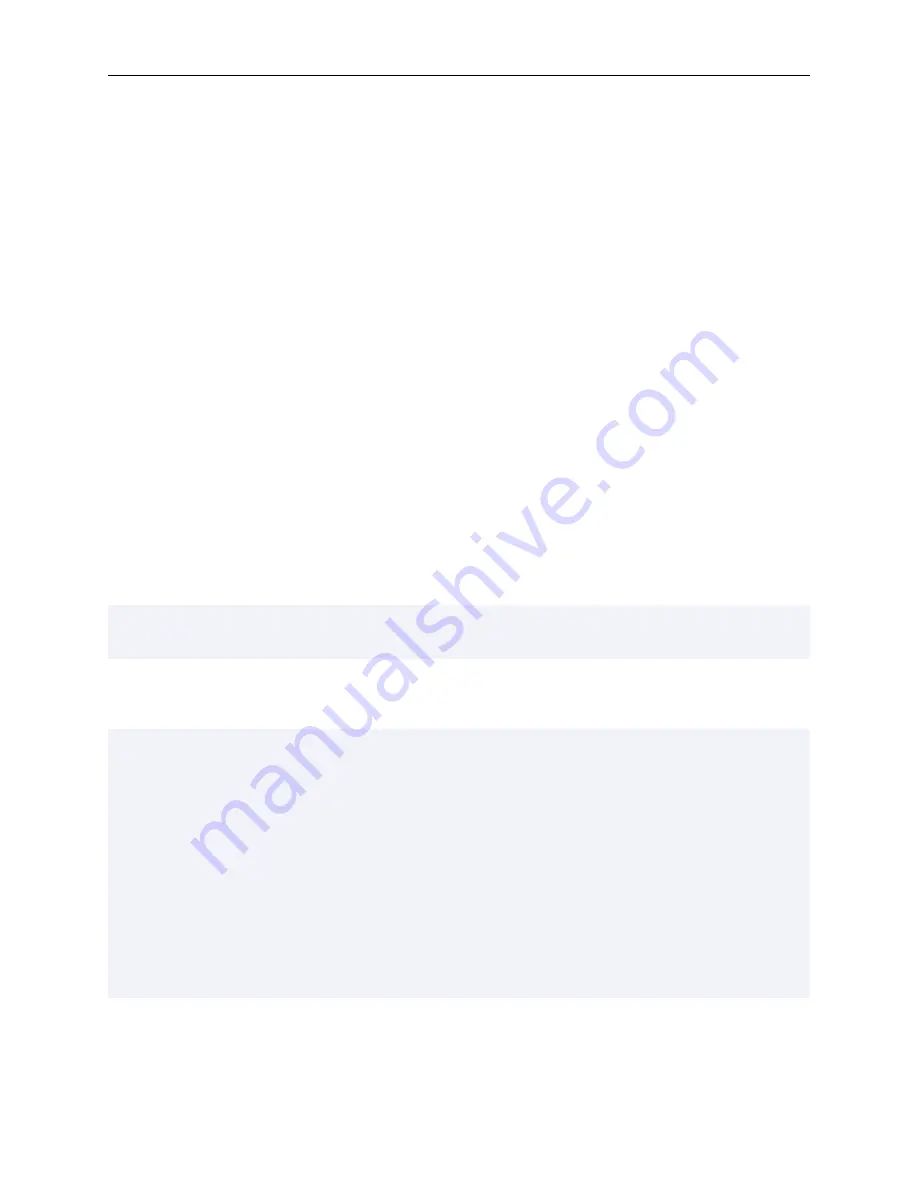
Using and understanding the Valgrind core: Advanced Topics
•
v.translate <address> [<traceflags>]
shows the translation of the block containing
address
with
the given trace flags. The
traceflags
value bit patterns have similar meaning to Valgrind’s
--trace-flags
option. It can be given in hexadecimal (e.g. 0x20) or decimal (e.g. 32) or in binary 1s and 0s bit (e.g. 0b00100000).
The default value of the traceflags is 0b00100000, corresponding to "show after instrumentation". The output of
this command always goes to the Valgrind log.
The additional bit flag 0b100000000 (bit 8) has no equivalent in the
--trace-flags
option. It enables tracing of
the gdbserver specific instrumentation. Note that this bit 8 can only enable the addition of gdbserver instrumentation
in the trace. Setting it to 0 will not disable the tracing of the gdbserver instrumentation if it is active for some other
reason, for example because there is a breakpoint at this address or because gdbserver is in single stepping mode.
3.3. Function wrapping
Valgrind allows calls to some specified functions to be intercepted and rerouted to a different, user-supplied function.
This can do whatever it likes, typically examining the arguments, calling onwards to the original, and possibly
examining the result. Any number of functions may be wrapped.
Function wrapping is useful for instrumenting an API in some way.
For example, Helgrind wraps functions in
the POSIX pthreads API so it can know about thread status changes, and the core is able to wrap functions in the
MPI (message-passing) API so it can know of memory status changes associated with message arrival/departure.
Such information is usually passed to Valgrind by using client requests in the wrapper functions, although the exact
mechanism may vary.
3.3.1. A Simple Example
Supposing we want to wrap some function
int foo ( int x, int y ) { return x + y; }
A wrapper is a function of identical type, but with a special name which identifies it as the wrapper for
foo
. Wrappers
need to include supporting macros from
valgrind.h
. Here is a simple wrapper which prints the arguments and
return value:
#include <stdio.h>
#include "valgrind.h"
int I_WRAP_SONAME_FNNAME_ZU(NONE,foo)( int x, int y )
{
int
result;
OrigFn fn;
VALGRIND_GET_ORIG_FN(fn);
printf("foo’s wrapper: args %d %d\n", x, y);
CALL_FN_W_WW(result, fn, x,y);
printf("foo’s wrapper: result %d\n", result);
return result;
}
To become active, the wrapper merely needs to be present in a text section somewhere in the same process’ address
space as the function it wraps, and for its ELF symbol name to be visible to Valgrind. In practice, this means either
45