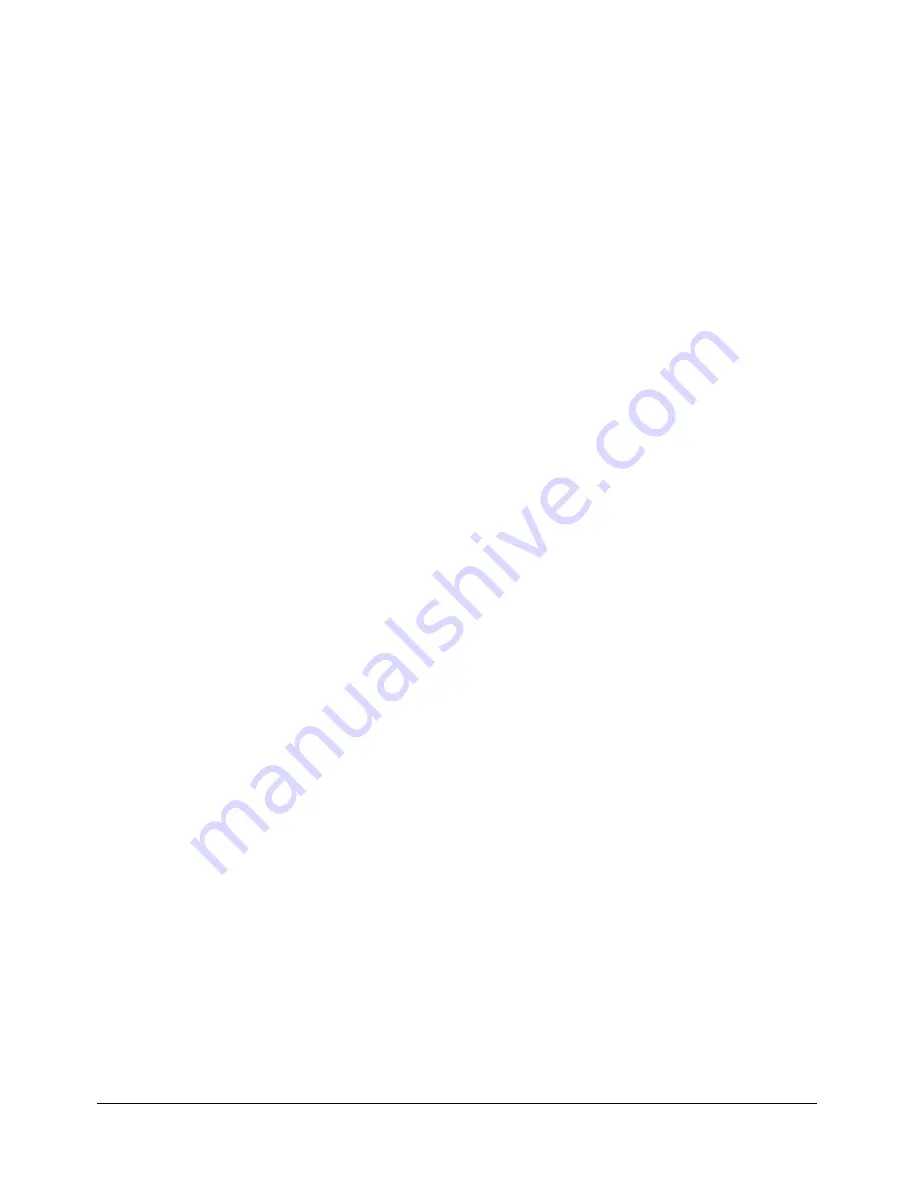
Creating user-defined functions
199
A simple CFScript example
The following example function adds the two arguments and returns the result:
<cfscript>
function Sum(a,b) {
var sum = a + b;
return sum;
}
</cfscript>
In this example, a single line declares the function variable and uses an expression to set it to the
value to be returned. This function can be simplified so that it does not use a function variable, as
follows:
function MySum(a,b) {Return a + b;}
You must always use curly braces around the function definition body, even if it is a single
statement.
Using the Arguments scope in CFScript
A function can have optional arguments that you do not have to specify when you call the
function. To determine the number of arguments passed to the function, use the following
function:
ArrayLen(Arguments)
When you define a function using CFScript, the function must use the Arguments scope to
retrieve the optional arguments. For example, the following SumN function adds two or more
numbers together. It requires two arguments and supports any number of additional optional
arguments. You can refer to the first two, required, arguments as
Arg1
and
Arg2
or as
Arguments[1]
and
Arguments[2]
. You must refer to the third, fourth, and any additional
optional arguments as
Arguments[3]
,
Arguments[4],
and so on.
function SumN(Arg1,Arg2) {
var arg_count = ArrayLen(Arguments);
var sum = 0;
var i = 0;
for( i = 1 ; i LTE arg_count; i = i + 1 )
{
sum = sum + Arguments[i];
}
return sum;
}
With this function, any of the following function calls are valid:
SumN(Value1, Value2)
SumN(Value1, Value2, Value3)
SumN(Value1, Value2, Value3, Value4)
and so on.
The code never uses the Arg1 and Arg2 argument variables directly, because their values are
always the first two elements in the Arguments array and it is simpler to step through the array.
Specifying Arg1 and Arg2 in the function definition ensures that ColdFusion generates an error if
you pass the function one or no arguments.
Summary of Contents for ColdFusion MX
Page 1: ...Developing ColdFusion MX Applications...
Page 22: ...22 Contents...
Page 38: ......
Page 52: ...52 Chapter 2 Elements of CFML...
Page 162: ......
Page 218: ...218 Chapter 10 Writing and Calling User Defined Functions...
Page 250: ...250 Chapter 11 Building and Using ColdFusion Components...
Page 264: ...264 Chapter 12 Building Custom CFXAPI Tags...
Page 266: ......
Page 314: ...314 Chapter 14 Handling Errors...
Page 344: ...344 Chapter 15 Using Persistent Data and Locking...
Page 349: ...About user security 349...
Page 357: ...Security scenarios 357...
Page 370: ...370 Chapter 16 Securing Applications...
Page 388: ...388 Chapter 17 Developing Globalized Applications...
Page 408: ...408 Chapter 18 Debugging and Troubleshooting Applications...
Page 410: ......
Page 426: ...426 Chapter 19 Introduction to Databases and SQL...
Page 476: ...476 Chapter 22 Using Query of Queries...
Page 534: ...534 Chapter 24 Building a Search Interface...
Page 556: ...556 Chapter 25 Using Verity Search Expressions...
Page 558: ......
Page 582: ...582 Chapter 26 Retrieving and Formatting Data...
Page 668: ......
Page 734: ...734 Chapter 32 Using Web Services...
Page 760: ...760 Chapter 33 Integrating J2EE and Java Elements in CFML Applications...
Page 786: ...786 Chapter 34 Integrating COM and CORBA Objects in CFML Applications...
Page 788: ......