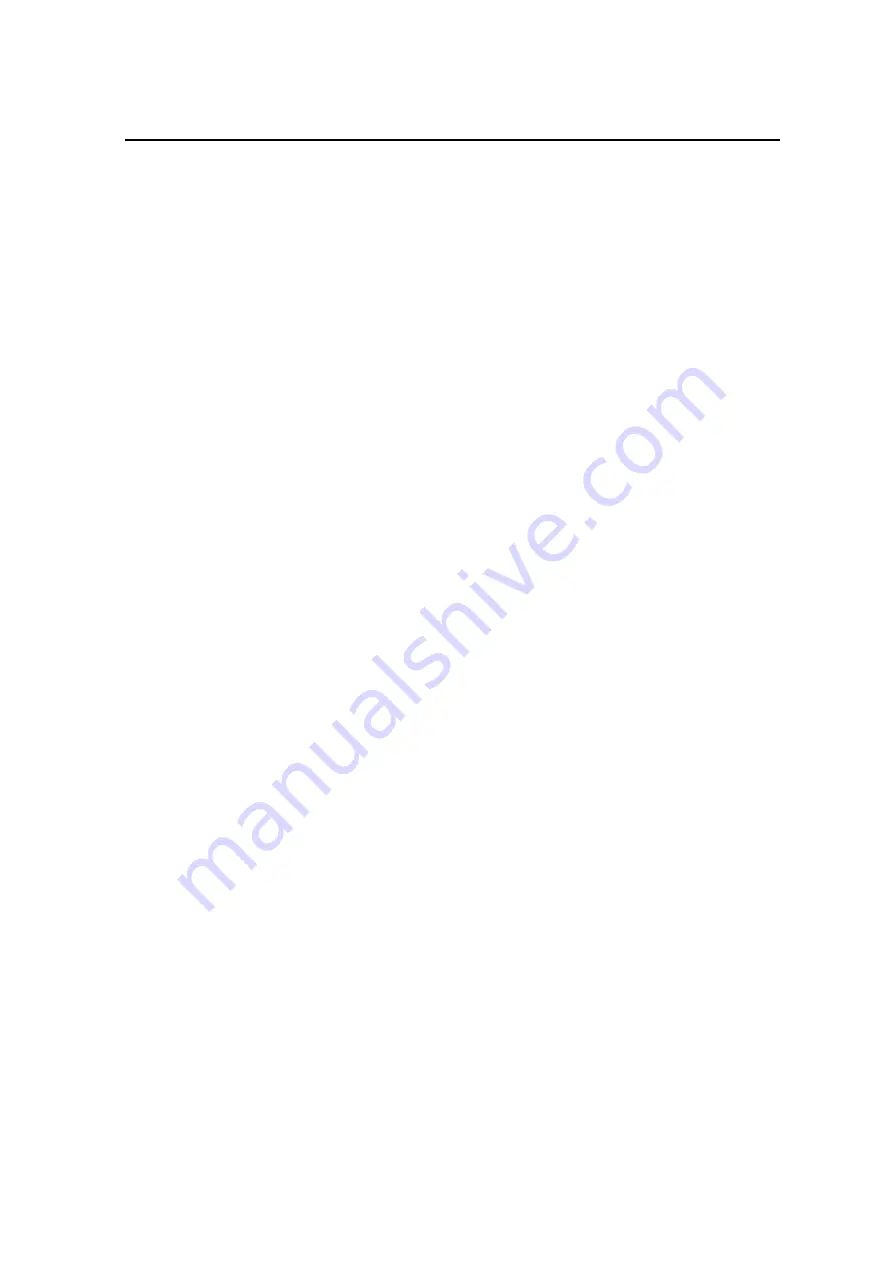
10 Hostmode
154
10.9.7
Example source code for CCITT CRC16 (HDLC)
Example program in Turbo PASCAL:
Program CCITT-CRC;
VAR
crc_table : ARRAY[0..255] OF Word; (* dynamically built up *)
crc : word;
Procedure CALC_CRC_CCITT( b : Byte);
BEGIN
crc := ((crc SHR 8) AND $ff) XOR crc_table[ (crc XOR b) AND $ff];
END;
Procedure InitCRC;
Var
index : Byte;
j, accu, Data : Word;
BEGIN
FOR index := 0 TO 255 DO
BEGIN (* build CRC-table *)
accu := 0;
Data := index;
FOR j := 0 TO 7 DO
BEGIN
IF Odd(Data XOR accu) THEN accu := (accu shr 1) XOR $8408
ELSE accu := (accu shr 1);
Data := Data shr 1;
END;
crc_table[index] := accu;
END; (* Build table *)
END;
Begin
InitCRC; (* Expand CRC-Table *)
crc := $ffff; (* Start-value of the CRC-Register *)
(* Calculating the CRC follows - for every input-byte
CALC_CRC_CCITT must be called once. *)
CALC_CRC_CCITT(04); (* Give input bytes in decimal here *)
CALC_CRC_CCITT(01);
CALC_CRC_CCITT(01);
CALC_CRC_CCITT(71);
CALC_CRC_CCITT(71);
(* and so on.... *)
CRC:=NOT(CRC); (* CRC is inverted at the end according to HDLC-protocol *)
(* in WORD CRC is contained the CRC value. When all bytes that should be