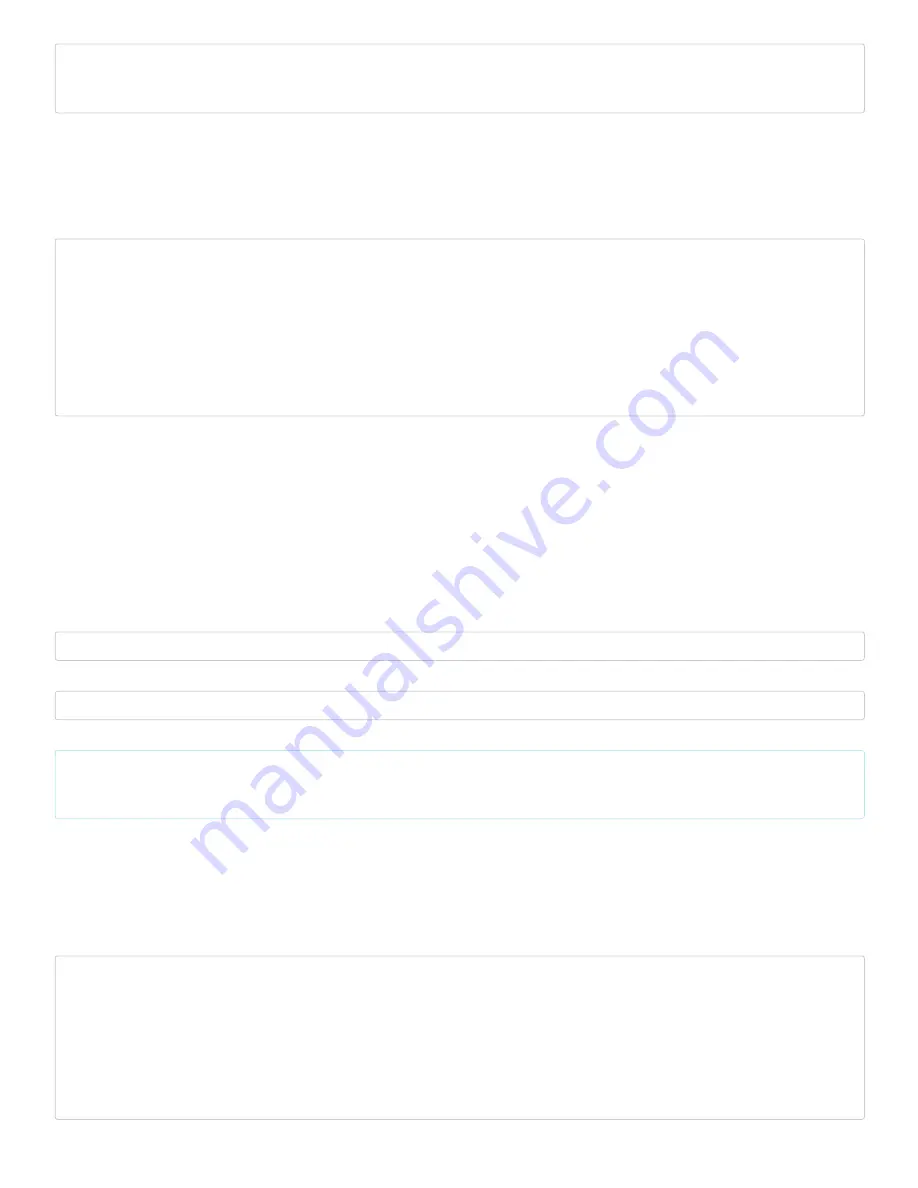
Press finger to Enroll #4
Remove finger
Press same finger again
Failed to capture second finger
Try enrolling a fingerprint by uploading the code and following the serial monitor’s output. To enroll more than one fingerprint, just reset the Arduino.
Example 3: Identifying w/ FPS_IDFinger.ino
The FPS_IDFinger.ino sketch checks to see if a finger is on the scanner. Once a finger has been placed on the scanner, it checks the fingerprint against any of the fingerprints
saved in the local database. You will be notified through the serial monitor if the fingerprint matches an ID, if the fingerprint is not found, or when it fails to read the fingerprint.
After checking and lifting your finger, it will request for another fingerprint to check.
Below is what you would expect when using this example:
Verified ID:0
Finger not found
Finger not found
Verified ID:0
Verified ID:0
Please press finger
Please press finger
Please press finger
Verified ID:2
Please press finger
Verified ID:2
Please press finger
Looking at the output, “Finger not found” usually means that: the fingerprint does not match any of the template IDs or when the the scanner is not able to clearly read the
fingerprint. If the finger has been enrolled, you would need to make sure that you place the fingerprint on the scanner just like when you scanned the finger.
Depending on what model you are using, make sure to change number of IDs in the condition statement. By default, the code uses 200 since the GT-511C3 can hold up to
200 fingerprint templates. If you are using the GT-511C1R, you would need to change the number to 20. Try testing the scanner with the code to see if the scanner is able to
read the fingerprints that were enrolled.
Software Serial w/ Other Microcontrollers
The demo code was originally designed for the ATmega328P on the Arduino Uno. If you were using it with ATmega2560 (i.e. Arduino Mega 2560) or ATmega32U4 (i.e.
Arduino Leonardo, Pro Micro 5V/16MHz, Pro Micro 3.3V/8Mhz, FioV3, etc.), you would need to re-configure the software serial pin definitions and adjust the connections. Not
all the pins can support change interrupts for a serial Rx pin depending on what Arduino microcontroller is used. For more information, try looking at the reference language
for the Software Serial library.
To use the FPS on an Arduino Mega 2560 or Arduino Leonardo, you would just need to comment out the line where it says:
SoftwareSerial fps(4, 5); // (Arduino SS_RX = pin 4, Arduino SS_TX = pin 5)
and uncomment out the line here:
SoftwareSerial fps(10, 11); // (Arduino SS_RX = pin 10, Arduino SS_TX = pin 11)
Once you change the code, make sure to rewire your connections to follow the pin definitions.
Caution: The FPS_GT511C3 library may not work for all microcontrollers using the Arduino IDE. As you move away from the ATmega328P family, you may need to
modify the code or port the library over to get it working. It would be easier and faster to just have an Atmega328P bootloaded with Arduino to handle the FPS code. To
use the fingerprint scanner, you could just write additional code to have the ATmega328P send serial data to the other microcontroller.
Firmware Overview
If you are interested, this section goes just a little further by looking briefly at the command protocol. We will be taking a quick look at the fingerprint scanner’s blink example
with an Arduino and how the command protocol functions based on the manual.
Verifying the Checksum Value
To verify the check sum for the command packet (command) or response packet (acknowledge), you would add the bytes of the command start codes, device id, parameter,
and command/response. Looking at the Arduino blink example, the serial monitor outputs:
FPS - Open
FPS - SEND: "55 AA 01 00 00 00 00 00 01 00 01 01"
FPS - RECV: "55 AA 01 00 00 00 00 00 30 00 30 01"
FPS - LED on
FPS - SEND: "55 AA 01 00 01 00 00 00 12 00 13 01"
FPS - RECV: "55 AA 01 00 00 00 00 00 30 00 30 01"
FPS - LED off
FPS - SEND: "55 AA 01 00 00 00 00 00 12 00 12 01"
FPS - RECV: "55 AA 01 00 00 00 00 00 30 00 30 01"
The example displays the packet structure as a multi-byte item represented as little endian. Breaking down the LED command to turn the LED OFF in hex, it is: