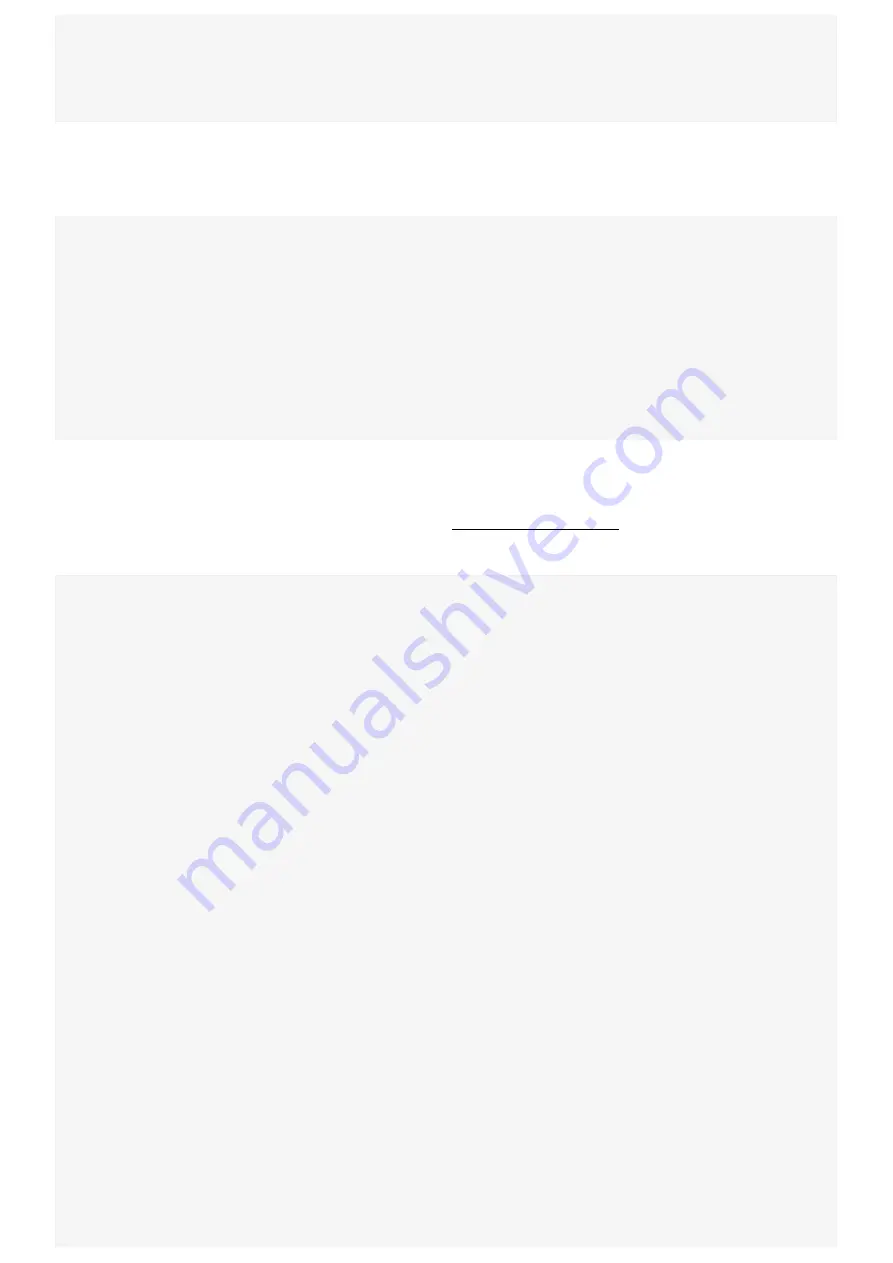
startSPIFFS();
// Start the SPIFFS and list all contents
startWebSocket();
// Start a WebSocket server
startMDNS();
// Start the mDNS responder
startServer();
// Start a HTTP server with a file read handler and an upload handler
}
As you can see, the setup is now much more condensed and gives a much better overview of what it's doing. To understand the
program, you don't have to know each individual step that is required to connect to a Wi-Fi network, it's enough to know that it will
connect to a Wi-Fi network, because that's what the startWiFi function does.
Loop
bool
rainbow
=
false
;
// The rainbow effect is turned off on startup
unsigned
long
prevMillis
=
millis
();
int
hue
=
0;
void
loop
() {
webSocket
.
loop
();
// constantly check for websocket events
server
.
handleClient
();
// run the server
ArduinoOTA
.
handle
();
// listen for OTA events
if
(rainbow) {
// if the rainbow effect is turned on
if
(
millis
()
>
prevMillis
+
32) {
if
(
++
hue
==
360)
// Cycle through the color wheel (increment by one degree every 32 ms)
hue
=
0;
setHue(hue);
// Set the RGB LED to the right color
prevMillis
=
millis
();
}
}
}
Same goes for the loop: most of the work is done by the first three functions that handle the WebSocket communication, HTTP
requests and OTA updates. When such an event happens, the appropriate handler functions will be executed. These are defined
elsewhere.
The second part is the rainbow effect. If it is turned on, it cycles through the color wheel and sets the color to the RGB LED.
If you don't understand why I use millis(), you can take a look at the Blink Without Delay example.
Setup functions
void
startWiFi() {
// Start a Wi-Fi access point, and try to connect to some given access points. Then wait for either an AP or STA
connection
WiFi
.
softAP
(ssid
,
password);
// Start the access point
Serial
.
(
"Access Point \""
);
Serial
.
(ssid);
Serial
.
println
(
"\" started\r\n"
);
wifiMulti
.
addAP(
"ssid_from_AP_1"
,
"your_password_for_AP_1"
);
// add Wi-Fi networks you want to connect to
wifiMulti
.
addAP(
"ssid_from_AP_2"
,
"your_password_for_AP_2"
);
wifiMulti
.
addAP(
"ssid_from_AP_3"
,
"your_password_for_AP_3"
);
Serial
.
println
(
"Connecting"
);
while
(wifiMulti
.
run
()
!=
WL_CONNECTED
&&
WiFi
.
softAPgetStationNum()
<
1) {
// Wait for the Wi-Fi to connect
delay
(250);
Serial
.
(
'.'
);
}
Serial
.
println
(
"\r\n"
);
if
(
WiFi
.
softAPgetStationNum()
==
0) {
// If the ESP is connected to an AP
Serial
.
(
"Connected to "
);
Serial
.
println
(
WiFi
.
SSID
());
// Tell us what network we're connected to
Serial
.
(
"IP address:\t"
);
Serial
.
(
WiFi
.
localIP
());
// Send the IP address of the ESP8266 to the computer
}
else
{
// If a station is connected to the ESP SoftAP
Serial
.
(
"Station connected to ESP8266 AP"
);
}
Serial
.
println
(
"\r\n"
);
}
void
startOTA() {
// Start the OTA service
ArduinoOTA
.
setHostname(OTAName);
ArduinoOTA
.
setPassword(OTAPassword);
ArduinoOTA
.
onStart([]() {
Serial
.
println
(
"Start"
);
digitalWrite
(LED_RED
,
0);
// turn off the LEDs
digitalWrite
(LED_GREEN
,
0);
digitalWrite
(LED_BLUE
,
0);
});
ArduinoOTA
.
onEnd([]() {
Serial
.
println
(
"\r\nEnd"
);
});
ArduinoOTA
.
onProgress([](
unsigned
int
progress
,
unsigned
int
total) {
Serial
.
printf
(
"Progress: %u%%\r"
,
(progress
/
(total
/
100)));
});
ArduinoOTA
.
onError
([](ota_error_t error) {
Serial
.
printf
(
"Error[%u]: "
,
error);
if
(error
==
OTA_AUTH_ERROR)
Serial
.
println
(
"Auth Failed"
);
else
if
(error
==
OTA_BEGIN_ERROR)
Serial
.
println
(
"Begin Failed"
);
else
if
(error
==
OTA_CONNECT_ERROR)
Serial
.
println
(
"Connect Failed"
);
else
if
(error
==
OTA_RECEIVE_ERROR)
Serial
.
println
(
"Receive Failed"
);
else
if
(error
==
OTA_END_ERROR)
Serial
.
println
(
"End Failed"
);
});
ArduinoOTA
.
begin
();
Serial
.
println
(
"OTA ready\r\n"
);
}
void
startSPIFFS() {
// Start the SPIFFS and list all contents
SPIFFS
.
begin
();
// Start the SPI Flash File System (SPIFFS)
Serial
.
println
(
"SPIFFS started. Contents:"
);
{
Dir dir
=
SPIFFS
.
openDir(
"/"
);