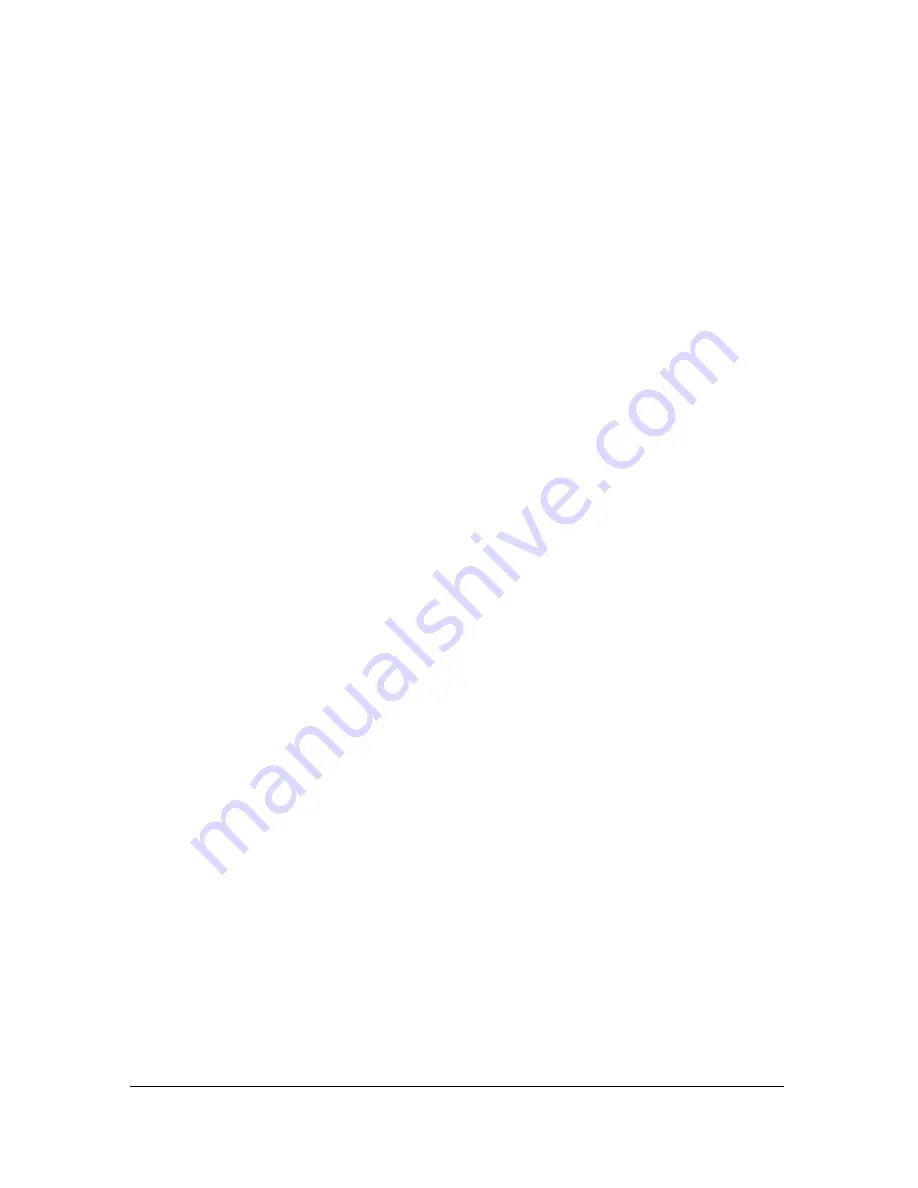
Statements
219
In the following example, the
finally
block is used to delete an ActionScript object,
regardless of whether an error occurred. Create a new AS file called Account.as.
class Account {
var balance:Number = 1000;
function getAccountInfo():Number {
return (Math.round(Math.random() * 10) % 2);
}
}
In the same directory as Account.as, create a new AS or FLA document and enter the
following ActionScript in Frame 1 of the Timeline:
import Account;
var account:Account = new Account();
try {
var returnVal = account.getAccountInfo();
if (returnVal != 0) {
throw new Error("Error getting account information.");
}
}
finally {
if (account != null) {
delete account;
}
}
The following example demonstrates a
try..catch
statement. The code within the
try
block is executed. If an exception is thrown by any code within the
try
block, control passes
to the
catch
block, which shows the error message in a text field using the
Error.toString()
method.
In the same directory as Account.as, create a new FLA document and enter the following
ActionScript in Frame 1 of the Timeline:
import Account;
var account:Account = new Account();
try {
var returnVal = account.getAccountInfo();
if (returnVal != 0) {
throw new Error("Error getting account information.");
}
trace("success");
}
catch (e) {
this.createTextField("status_txt", this.getNextHighestDepth(), 0, 0, 100,
22);
status_txt.autoSize = true;
status_txt.text = e.toString();
}
Summary of Contents for FLASHLITE2 ACTIONSCRIPT-LANGUAGE
Page 1: ...Flash Lite 2 x ActionScript Language Reference...
Page 22: ...22 Contents...
Page 244: ...244 ActionScript language elements...
Page 760: ...760 ActionScript classes...