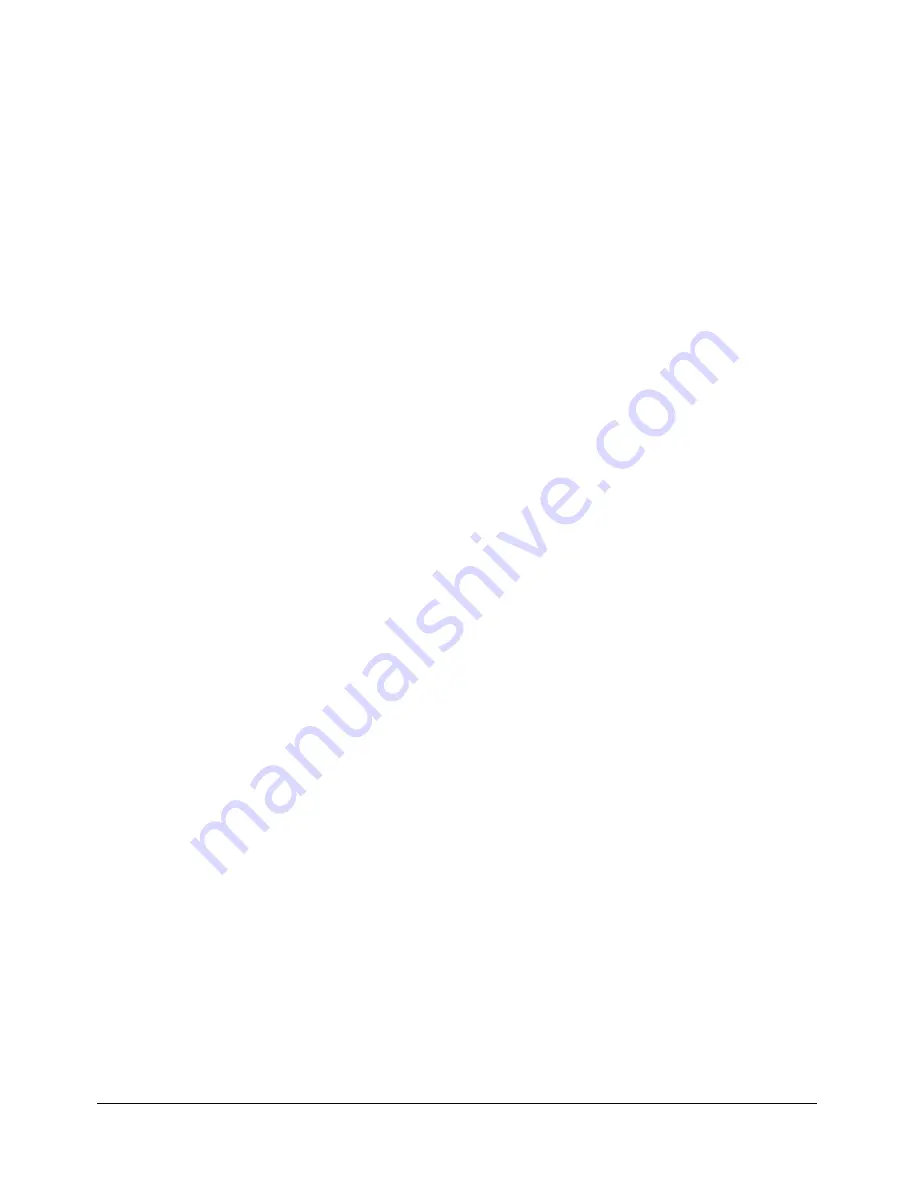
Binding properties to a custom component
31
Defining getters and setters
The recommended way of exposing properties in your class file is with a pair of getters and setters.
These functions must be public. The advantage of getters and setters is that you can calculate the
return value in a single place and trigger events when the variable changes.
You define getter and setter functions using the get and set method properties within a class
definition block.
The following example declares a getter and setter for the
myName
variable:
private var _myName:String = "Fred";
public function get myName():String {
return _myName;
}
public function set myName(name:String) {
_myName = name;
}
The local value of
_myName
is private, while the getter and setter methods are public.
In getters and setters, you cannot use the same name of the property in the function name. As a
result, you should use some convention, such as prefixing the member name with an underscore
character (_).
Binding properties to a custom component
Most properties should be bindable so that developers can use the contents of your component as
parameters to a web service call or to update the UI. The simplest form of binding is to use the
curly braces ({ }) syntax that binds a property of a control to another control’s property. In the
component’s ActionScript class, you can declare a variable as follows:
var myName:String = "Fred";
In your MXML file, you then bind the value of a control’s property to the component’s property,
as the following example shows:
<MyComponent id="myComp1" name="Fred" />
<mx:TextArea
text="{myComp1.myName}"
/>
Although it is sometimes useful, this simple binding technique does not fully take advantage of
the capabilities of binding. If the user changes the value of the MyComponent
myName
property,
the TextArea component is not notified. To keep the two synchronized, the MXML author must
write code that stores and periodically compares the two values.
With a little modification, the component’s class file can support dynamic binding so that
whenever the component’s property changes, the Flex control reflects that change.
To be dynamically bindable, a property must dispatch an event when it is changed. The main
property of a component often dispatches either a
change
or
click
event when it is accessed, but
you can use any event.