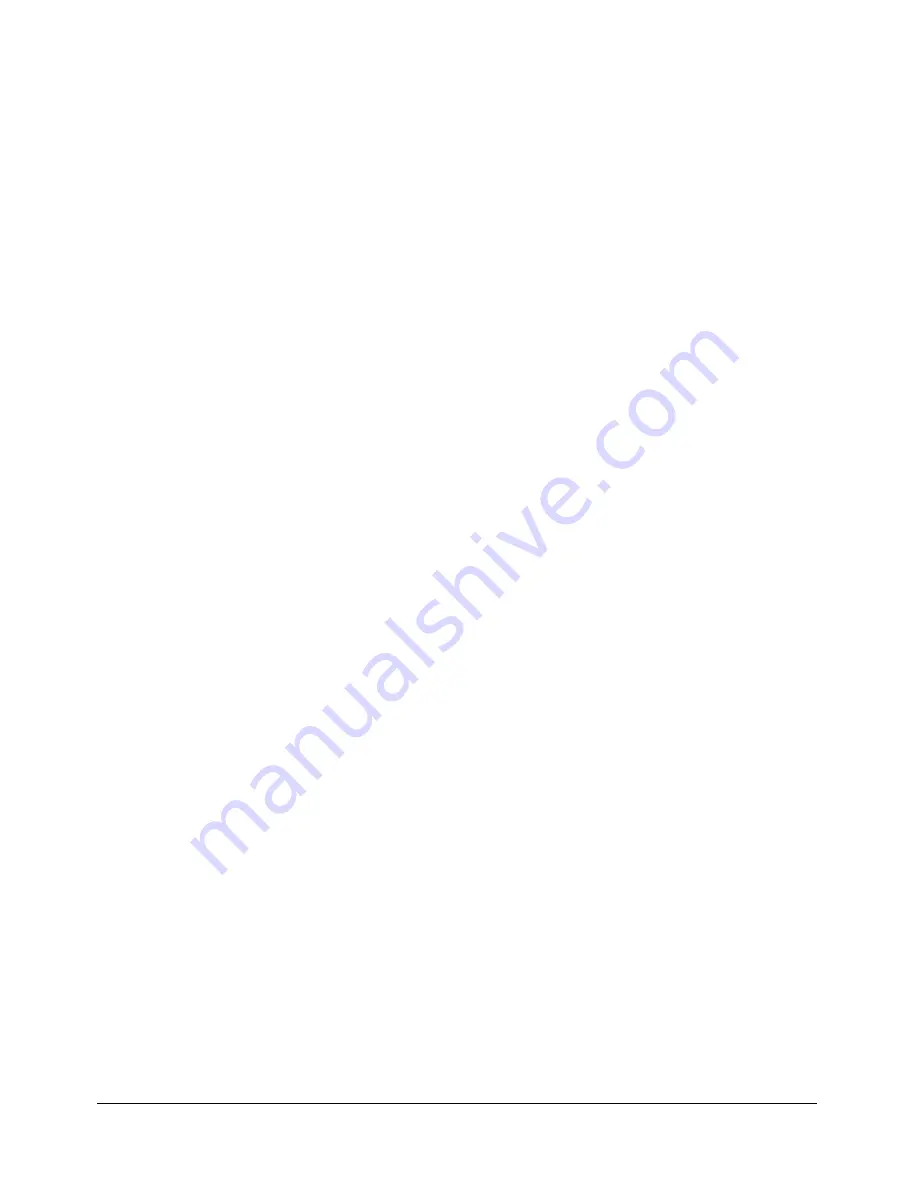
Using the ModalText example
73
•
Always implement an
init()
method and call the
super.init()
method, but otherwise keep
the component as lightweight as possible.
•
Use the
invalidate()
and
invalidateStyle()
methods to invoke the
draw()
method
instead of calling the
draw()
method explicitly.
Using the ModalText example
The following code example implements the class definition for the ModalText component. This
is a sample custom component that is included in the Flex installation in the
flex_install_dir
/
resources/flexforflash directory. The ModalText.zip file contains ModalText.fla, a file that defines
the symbols for the ModalText component necessary to generate the SWC file. This ZIP file also
contains ModalText.as, which is also provided here.
The ModalText component is a text input whose default is the noneditable mode, but you can
switch to editable mode by clicking its button.
Save the following code to the file ModalText.as, and then generate the SWC file using the
FLA file:
// Import all necessary classes.
import mx.core.UIComponent;
import mx.controls.SimpleButton;
import mx.controls.TextInput;
// Modal text sends a change event when the text is changed.
[Event("change")]
/*** a) Extend UIComponent. ***/
class ModalText extends UIComponent {
/*** b) Specify symbolName, symbolOwner, className. ***/
static var symbolName:String = "ModalText";
static var symbolOwner:Object = ModalText;
// className is optional and used for determining default styles.
var className:String = "ModalText";
/*** c) Specify clipParameters, which are the properties you want to set
before the call to init() and createChildren() ***/
static var clipParameters = { text:1, labelPlacement: 1 };
/***d) Implement constructObject2(), which is effectively the constructor
for this class. ***/
function constructObject2(o:Object):Void
{
super.constructObject2(o);
applyProperties(o, ModalText.clipParameters);
}
/*** e) Implement init(). ***/
function init():Void
{
// Set up initial values based on clipParameters, if any (there are none