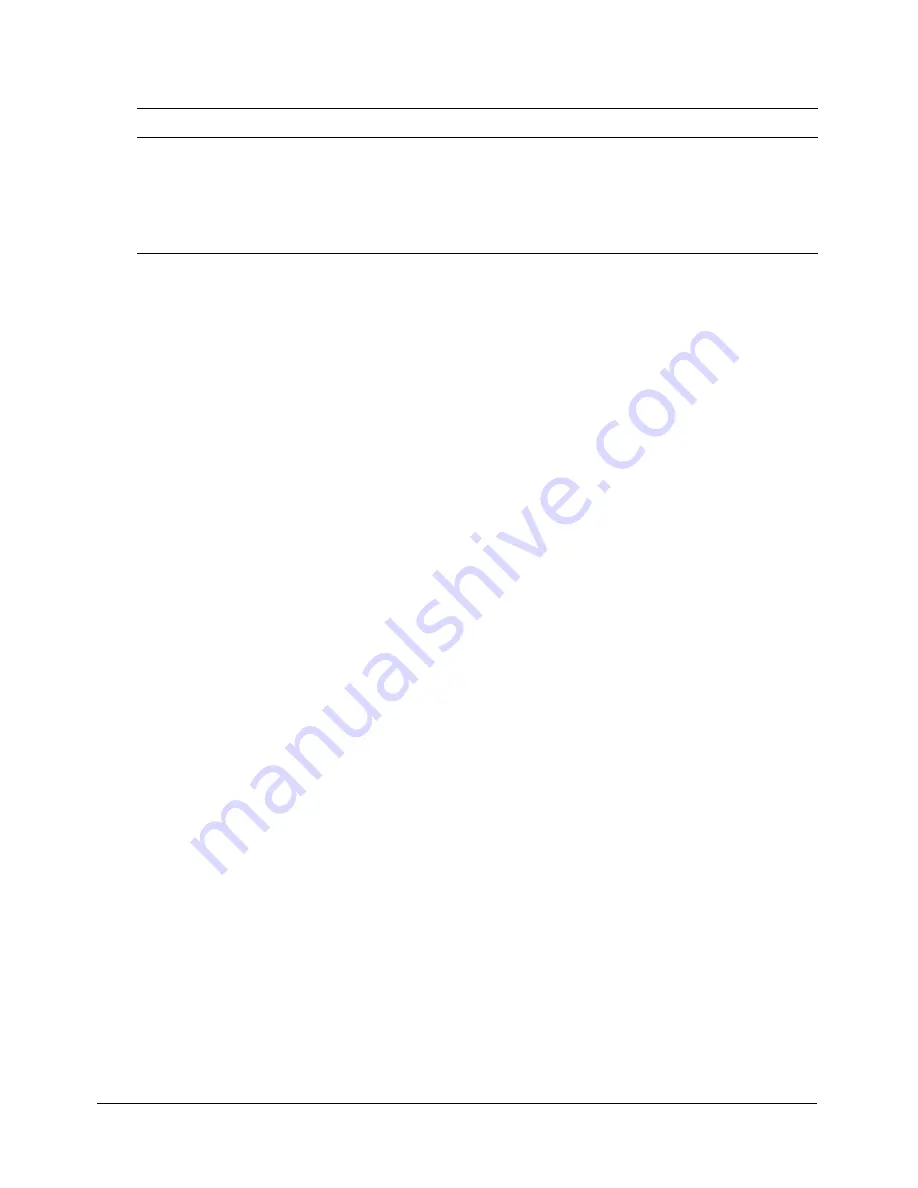
Writing the component’s ActionScript code
55
The following table describes the arguments:
To call the
createClassObject()
method, you must know what those children are (for example,
a border or a button that you always need), because you must specify the name and type of the
object, plus any initialization parameters in the call to
createClassObject()
.
The following example calls the
createClassObject()
method to create a new Label object for
use inside a component:
up_mc.createClassObject(Label, "label_mc", 1); // Create a label in the holder
You set properties in the call to the
createClassObject()
method by adding them as part of the
initObject
argument. The following example sets the value of the
label
property:
form.createClassObject(CheckBox, "cb", 0, {label:"Check this"});
The following example creates TextInput and SimpleButton components:
function createChildren():Void {
if (text_mc == undefined)
createClassObject(TextInput, "text_mc", 0, { preferredWidth: 80,
editable:false });
text_mc.addEventListener("change", this);
text_mc.addEventListener("focusOut", this);
if (mode_mc == undefined)
createClassObject(SimpleButton, "mode_mc", 1, { falseUpSkin:
modeUpSkinName, falseOverSkin: modeOverSkinName, falseDownSkin:
modeDownSkinName });
mode_mc.addEventListener("click", this);
}
If your component is a container, and you do not know exactly which children it contains, call the
createComponents()
method. This method creates all child objects. For more information on
using the
createComponents()
method, see
Developing Flex Applications
.
At the end of the
createChildren()
method, call the necessary invalidate methods
(
invalidate()
,
invalidateSize()
, or
invalidateLayout()
) to refresh the screen. For more
information, see
“About invalidation” on page 68
.
Implementing the commitProperties() method
Flex calls the
commitProperties()
method before it calls the
measure()
method. It provides a
chance to set variables that are used by the
measure()
method after the constructor has finished
and MXML attributes have been applied.
Argument
Type
Description
className
Object
The name of the class.
instanceName
String
The name of the instance.
depth
Number
The depth for the instance.
initObject
Object
The object that contains the initialization properties.