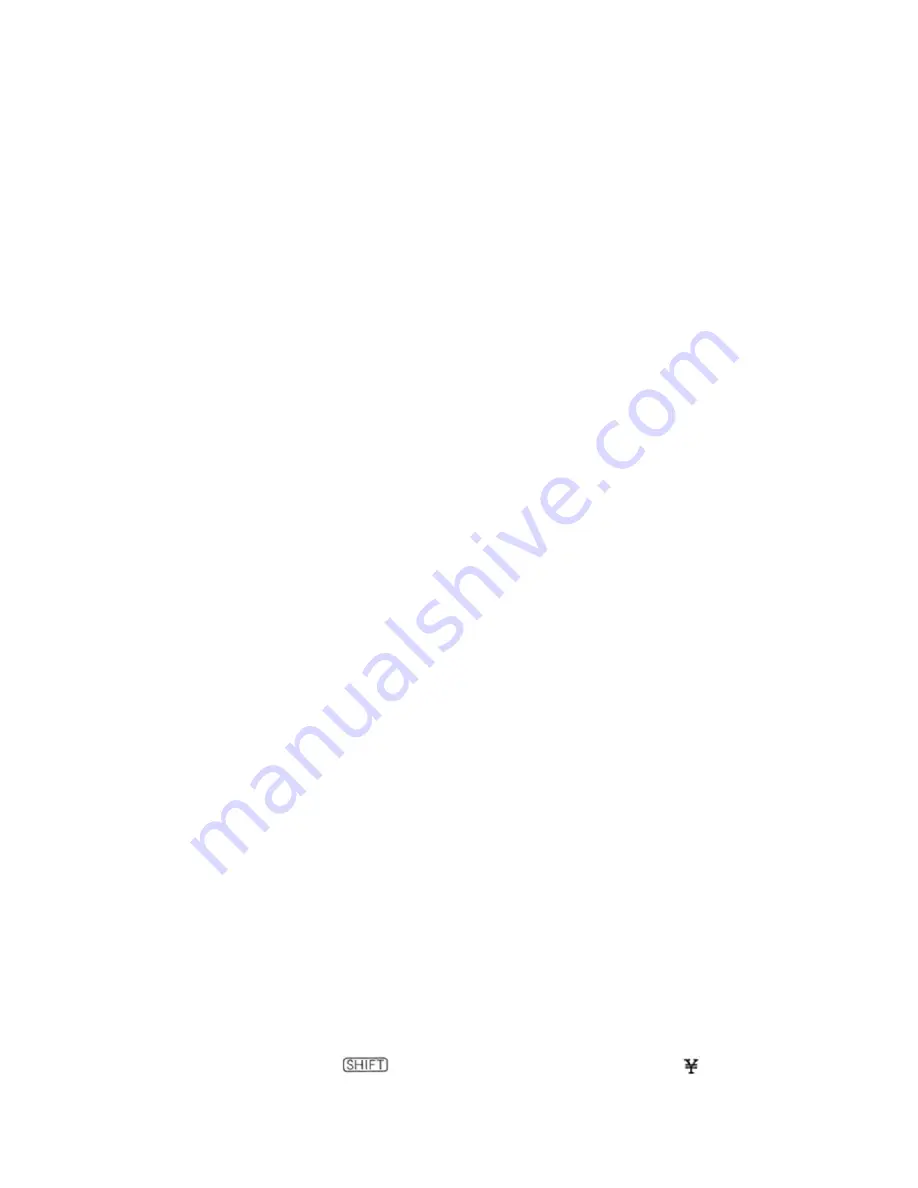
SHARP PC-G850V(S) User Manual -
Appendix A: 11-Pin Interface
15
The following C-program implements these requirements:
1 #define BOOL char
2 #define TRUE 1
3 #define FALSE 0
4 #define BTN 0x02
5
6 char BTNstate = 0;
7 char LEDstate = 0;
9
10 BOOL setupPIO() {
11 if(!fopen("pio","a+")) {
12 printf("can't open port\n");
13 return FALSE;
14 }
15 pioset(BTN);
16 return TRUE;
17 }
19
20 BOOL pressed() {
21 BOOL rtn=FALSE;
22 char btn;
23 btn=pioget()&BTN;
24 if(btn && BTNstate==0)
25 rtn=TRUE;
26 BTNstate=btn;
27 return rtn;
28 }
29
30 toggleLED() {
31 LEDstate=!LEDstate;
32 printf("LED=%x\n",LEDstate);
33 pioput(LEDstate);
34 }
39
100 main() {
101 printf("PIO test\n");
102 if(!setupPIO())
103 abort();
104 while(TRUE) {
105 if(pressed()){
106 printf("button pressed\n");
107 toggleLED();
108 }
109 }
110 }
To enter the symbol '\' press
-G in TEXT-Mode. It is displayed as
.