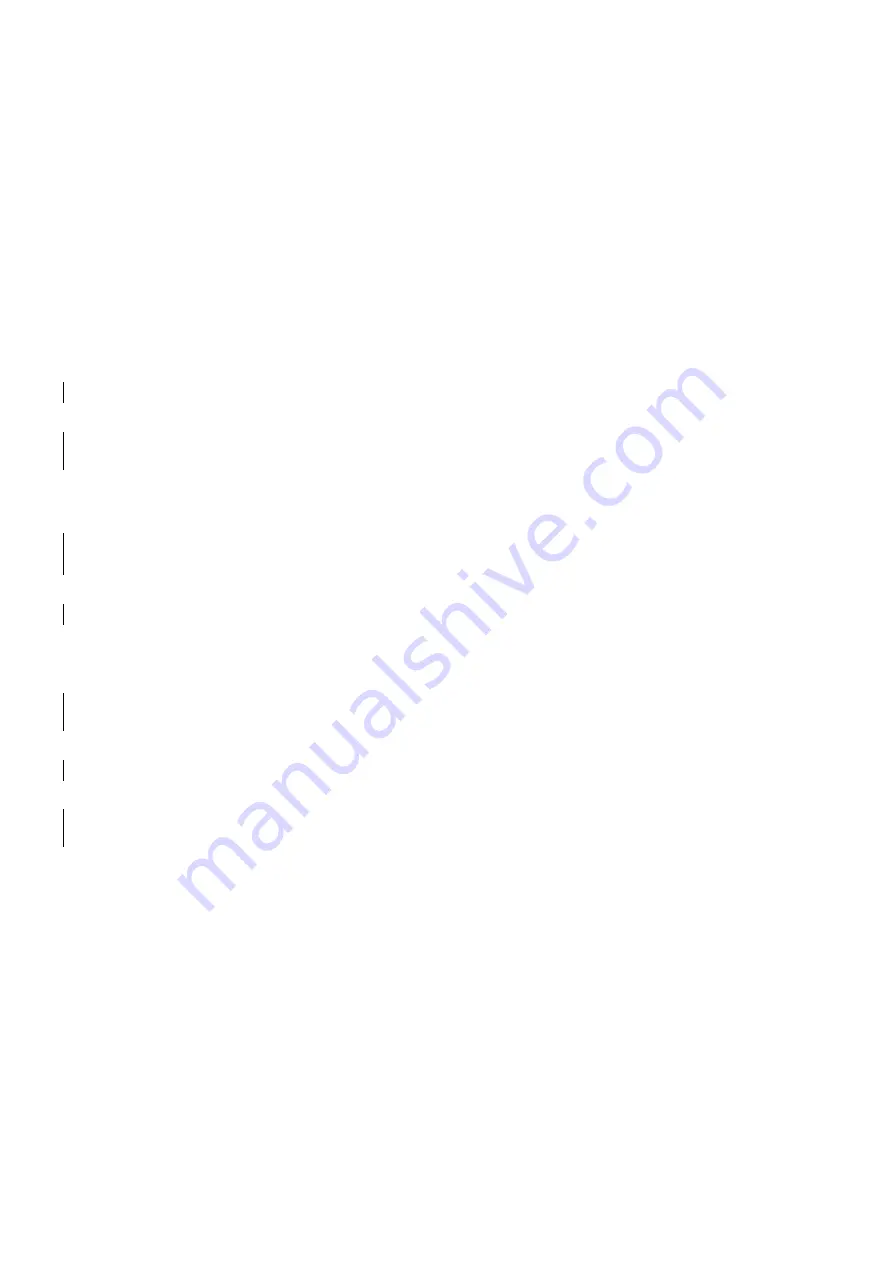
Programming and Operating Manual (Milling)
106
6FC5398-4DP10-0BA1, 01/2014
Programming/data types
DEF BOOL varname1
;Boolean type, values: TRUE (=1), FALSE (=0)
DEF CHAR varname2
;Char type, 1 ASCII code character: "a", "b", ...
;Numerical code value: 0 ... 255
DEF INT varname3
;Integer type, integer values, 32 bit value range:
;-2 147 483 648 t2 147 483 647 (decimal)
DEF REAL varname4
;Real type, natural number (like arithmetic parameter R),
;Value range: ±(0.000 0001 ... 9999 9999)
;(8 decimal places, arithmetic sign and decimal point) or
;Exponential notation: ± (10 to power of -300 ... 10 to power of +300)
DEF STRING[string length] varname41
; STRING type, [string length]: Maximum number of characters
Each data type requires its own program line. However, several variables of the same type can be defined in one line.
Example:
DEF INT PVAR1, PVAR2, PVAR3=12, PVAR4
;4 type INT variables
Example for STRING type with assignment:
DEF STRING[12] PVAR="Hello"
; Define variable PVAR with a maximum of 12
characters and assign string "Hello"
Fields
In addition to the individual variables, one or two-dimensional fields of variables of these data types can also be defined:
DEF INT PVAR5[n]
;One-dimensional field, type INT, n: integer
DEF INT PVAR6[n,m]
;Two-dimensional field, type INT, n, m: integer
Example:
DEF INT PVAR7[3]
;Field with 3 elements of the type INT
Within the program, the individual field elements can be reached via the field index and can be treated like individual
variables. The field index runs from 0 to a small number of the elements.
Example:
N10 PVAR7[2]=24
;The third field element (with index 2) is assigned the value
24.
Value assignment for field with SET instruction:
N20 PVAR5[2]=SET(1,2,3)
;After the 3rd field element, different values are assigned.
Value assignment for field with REP instruction:
N20 PVAR7[4]=REP(2)
;After field element [4] - all are assigned the same value,
here 2.
8.13.3
Reading and writing PLC variables
Functionality
To allow rapid data exchange between NC and PLC, a special data area exists in the PLC user interface with a length of 512
bytes. In this area, PLC data are compatible in data type and position offset. In the NC program, these compatible PLC
variables can be read or written.
To this end, special system variables are provided:
$A_DBB[n]
;Data byte (8-bit value)
$A_DBW[n]
;Data word (16-bit value)
$A_DBD[n]
;Data double-word (32-bit value)
$A_DBR[n]
;REAL data (32-bit value)
"n" stands here for the position offset (start of data area to start of variable) in bytes