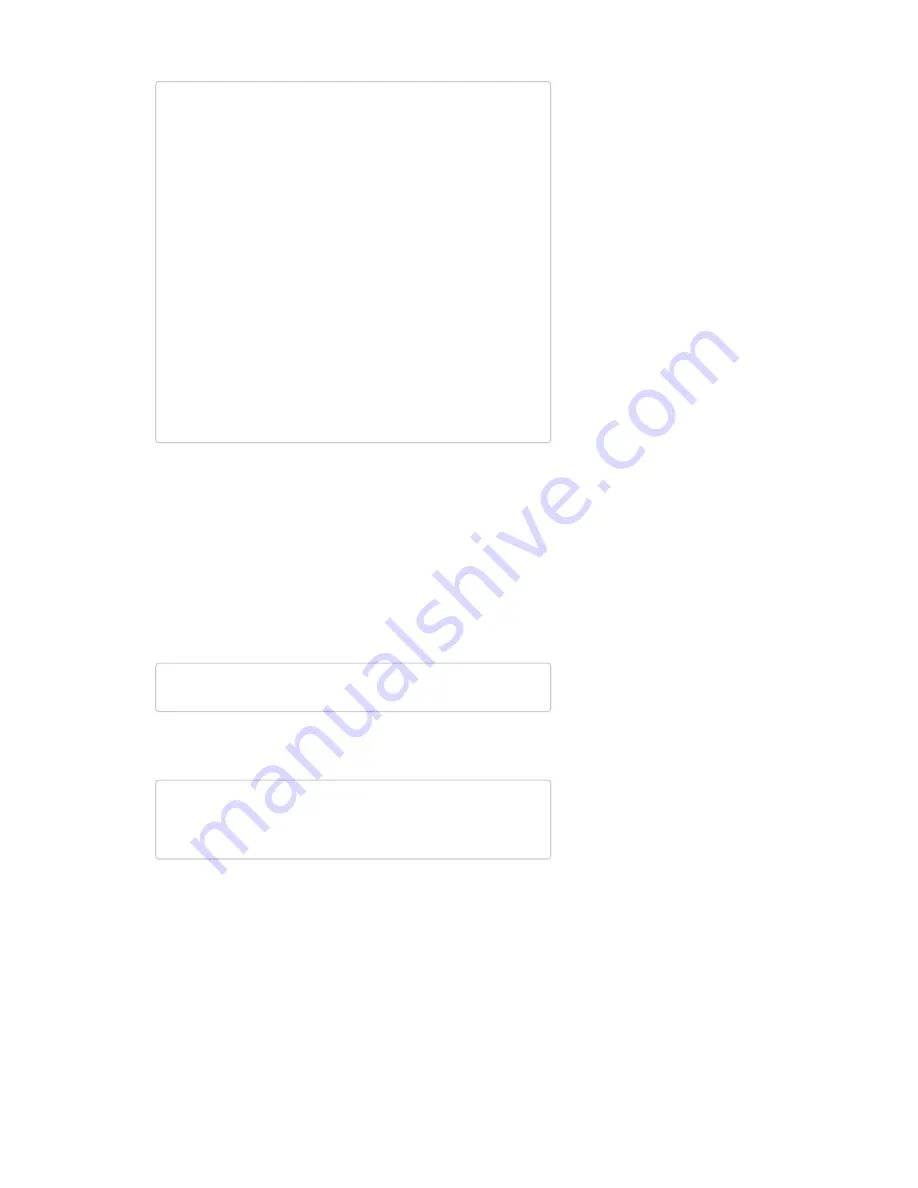
void loop()
{
// Measure each key press using capacitive sensing
measureKeys();
// Select one of three octaves based on position of potentio
meter
octave = readPot();
// Play the note corresponding to the key press and octave
// Higher pitch keys have priority if there were multiple pr
esses
playKeyPress();
// Play melody and turn on LED if the button has been presse
d
if (buttonPressed())
{
digitalWrite(led,HIGH);
playMelody();
}
}
Each time through the loop, we first measure each key press with
capacitive sensing to detect which ones are being pressed. We then check
the potentiometer to select which octave of notes we will play. We then play
the key press. If two or more keys are being pressed, we play the higher
frequency one by default. Finally, we check if the button is pressed, and, if it
is, we play a pre-programmed melody that’s stored in the
notes
array.
Feel free to dig into the full source code to view each loop functions'
implementation to understand how they work. We’ll go over a few of the
most important details here.
In the
measureKeys()
function, the most important line to understand is the
following:
keys[i] = CapSensors[i].capacitiveSensor(5); // 5 samples per
key
For each key, this takes 5 capacitive touch readings, averages them, and
then stores them in our
keys
array. These values are then used afterward
in the
playKeyPress()
function like this:
if (keys[12] > threshold)
{
tone(spkr,NOTE_C5 * octave,30);
}
Each key is checked to see if its value is greater than the set
threshold
. If
it is, we use Arduino’s built-in
tone()
function to play the corresponding
note for 30 milliseconds. Since the if statements check the keys from high
to low pitch, higher pitch keys take priority. Only one note is ever played at
a time.
The variable
spkr
is simply the pin to the on-board speaker. For the high C
(right most note on the board),
NOTE_C5
is the note/frequency to be played
through the speaker by default. The variable
octave
can be set to .5, 1.0,
or 2.0 depending on the position of the potentiometer that was read by the
readPot()
function. This value will be multiplied by the default
NOTE_C5
value (found in
pitches.h
), and the speaker will play the note
corresponding to the frequency just calculated.
Page 7 of 8