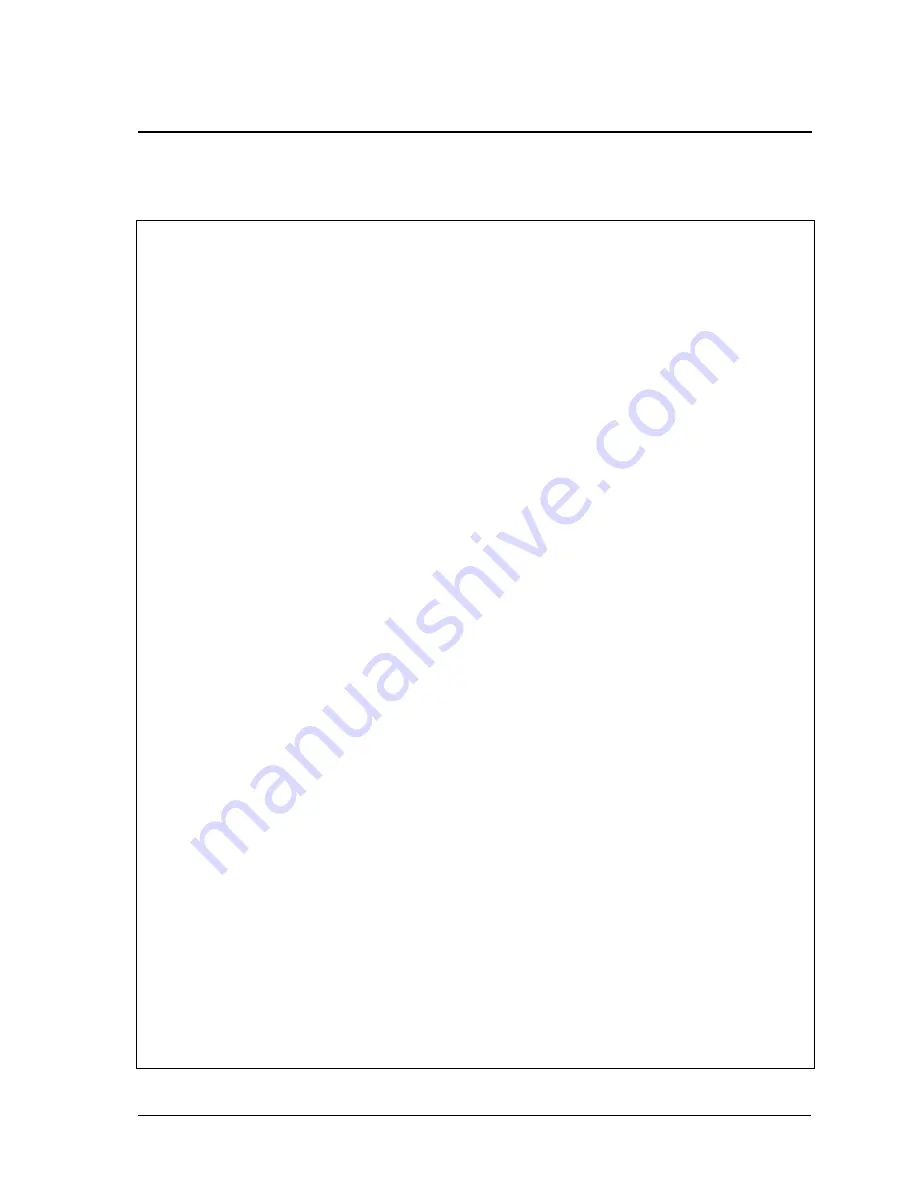
RP Series
March 2011
51
13.2.1. Serial Communication Test Program
The following sample program written in Quick-BASIC can be used to check communication
to the RP Series AC source over the RS232C serial interface. The interface is optional and
must be installed for this to work.
'California Instruments P Series RS232C Communication Demo Program
'(c) 1996 Copyright California Instruments, All Rights Reserved
'
'This program is for demonstration purposes only and is not to be
'used for any commercial application
'================================================================
'Function and Subroutine Declarations
DECLARE FUNCTION retstring$ ()
'================================================================
'MAIN PROGRAM CODE
'================================================================
'OPEN COM2. Replace with COM1, COM3 or COM4 for Com port used
'The input and output buffers are set to 2K each although
'this is not required for most operations.
OPEN "COM2:9600,n,8,1,BIN,LF,TB2048,RB2048" FOR RANDOM AS #1 LEN = 1
CLS
PRINT "**** P SERIES INTERACTIVE MODE ****"
'Enter and endless loop to accept user entered commands
DO
INPUT "Enter Command ('quit' to exit)--> ", cmd$
IF cmd$ <> "QUIT" AND cmd$ <> "quit" THEN
IF LEN(cmd$) > 0 THEN
PRINT #1, cmd$ + CHR$(10);
END IF
IF INSTR(cmd$, "?") THEN
PRINT "AC Source response = " + retstring$
END IF
'Check for Errors after each command is issued
PRINT #1, "*ESR?" + CHR$(10);
'Mask off bits 5,4,3,2 only. Other bits are not used.
esr% = VAL(retstring$) AND 60
'Process esr% value for error bits
IF esr% AND 4 THEN
PRINT "*** Query Error Reported by AC Source ***"
END IF
IF esr% AND 8 THEN
PRINT "*** Instrument Dependent Error Reported by AC Source ***"
END IF
IF esr% AND 16 THEN
PRINT "*** Command Execution Error Reported by AC Source ***"
END IF
IF esr% AND 32 THEN
PRINT "*** Command Syntax Error Reported by AC Source ***"
END IF
'Clear ERR. -XXX Message from front panel if any error occured
IF esr% <> 0 THEN
PRINT #1, "*CLS" + CHR$(10);
END IF
END IF
LOOP UNTIL cmd$ = "QUIT" OR cmd$ = "quit"
'Close COM port on exit
CLOSE #1
END
'================================================================
FUNCTION retstring$
'This function returns a response string from the P Series
'AC power source. The QBasic statement LINE INPUT cannot be used
'as the P Series does not return a CR <13> after a response
'message. The LINE INPUT function waits for a CR before
'returning a string. The P Series returns a LF <10> instead
'so we need to poll each returned character for a LF to