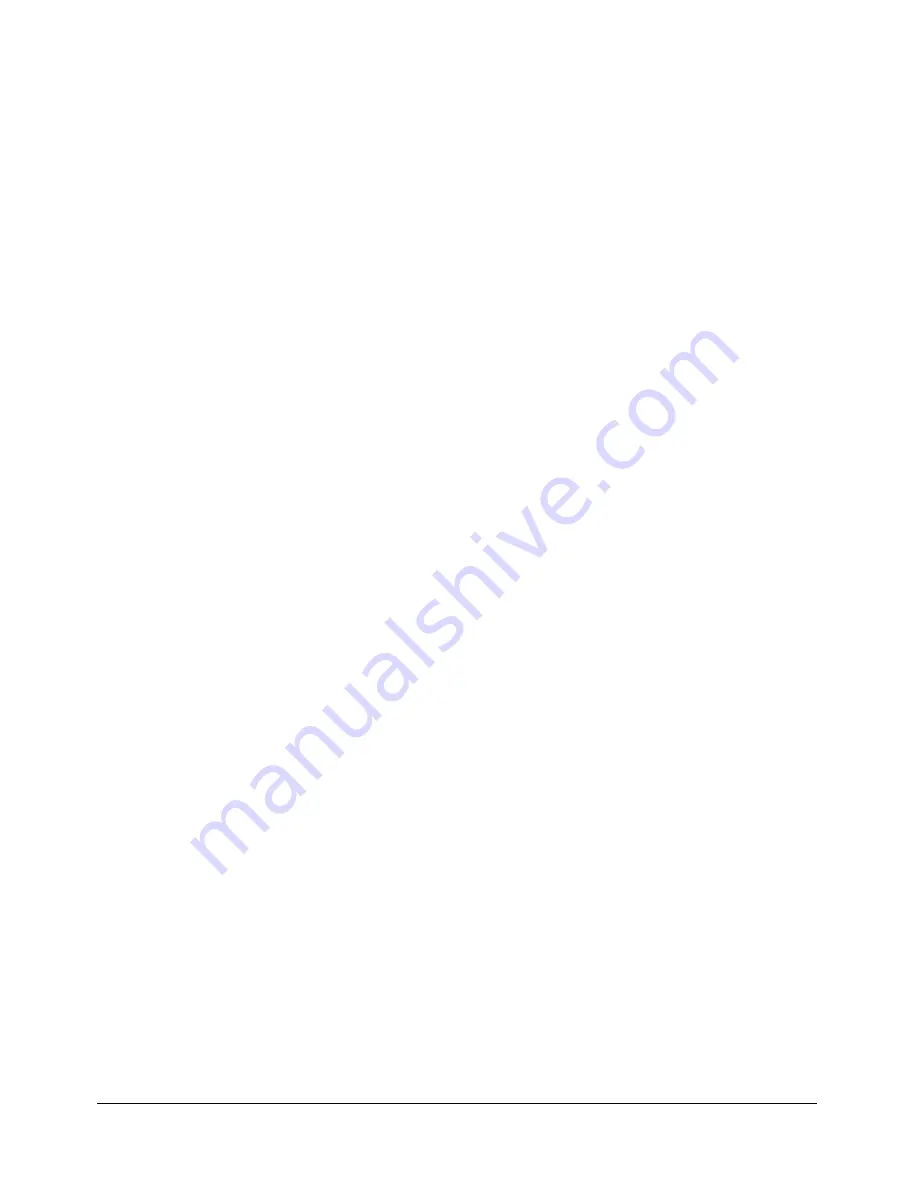
Events, messages, and handlers
37
For example, consider that you wrote a custom sprite method named
jump()
that takes a single
integer as a parameter, and you placed the method in a behavior. When you call
jump()
from a
sprite object reference, the handler must also include a parameter that represents the script object
reference, and not just the single integer. In this case, the implied parameter is represented by the
keyword
me
, but any term will work.
-- Lingo syntax
myHeight = sprite(2).jump(5)
on jump(me,a)
return a + 15 -- this handler works correctly, and returns 20
end
on jump(a)
return a + 15 -- this handler does not work correctly, and returns 0
end
You can also use expressions as values. For example, the following statement substitutes
3+6
for
a
and
8>2
(or
1
, representing
TRUE
) for
b
, and would return
10
:
-- Lingo syntax
mySum = addThem(3+6, 8>2)
In Lingo, each handler begins with the word
on
followed by the message that the handler should
respond to. The last line of the handler is the word
end
. You can repeat the handler’s name after
end
, but this is optional.
In JavaScript syntax, each handler begins with the word
function
followed by the message that
the handler should respond to. The statements that comprise the handler are surrounded by
opening and closing brackets, as are all JavaScript syntax functions.
Returning results from handlers
Often, you want a handler to report some condition or the result of some action.
To return results from a handler:
•
Use the keyword
return
to have a handler report a condition or the result of an action. For
example, the following
findColor
handler returns the current color of sprite 1:
-- Lingo syntax
on findColor
return sprite(1).foreColor
end
// JavaScript syntax
function findColor() {
return(sprite(1).foreColor);
}
You can also use the keyword
return
by itself to exit from the current handler and return no
value. For example, the following
jump
handler returns nothing if the
aVal
parameter equals 5;
otherwise, it returns a value.
-- Lingo syntax
on jump(aVal)
if aVal = 5 then return
aVal = aVal + 10
return aVal
end
Summary of Contents for DIRECTOR MX 2004-DIRECTOR SCRIPTING
Page 1: ...DIRECTOR MX 2004 Director Scripting Reference...
Page 48: ...48 Chapter 2 Director Scripting Essentials...
Page 100: ...100 Chapter 4 Debugging Scripts in Director...
Page 118: ...118 Chapter 5 Director Core Objects...
Page 594: ...594 Chapter 12 Methods...
Page 684: ...684 Chapter 14 Properties See also DVD...
Page 702: ...702 Chapter 14 Properties See also face vertices vertices flat...
Page 856: ...856 Chapter 14 Properties JavaScript syntax sprite 15 member member 3 4...
Page 1102: ...1102 Chapter 14 Properties...