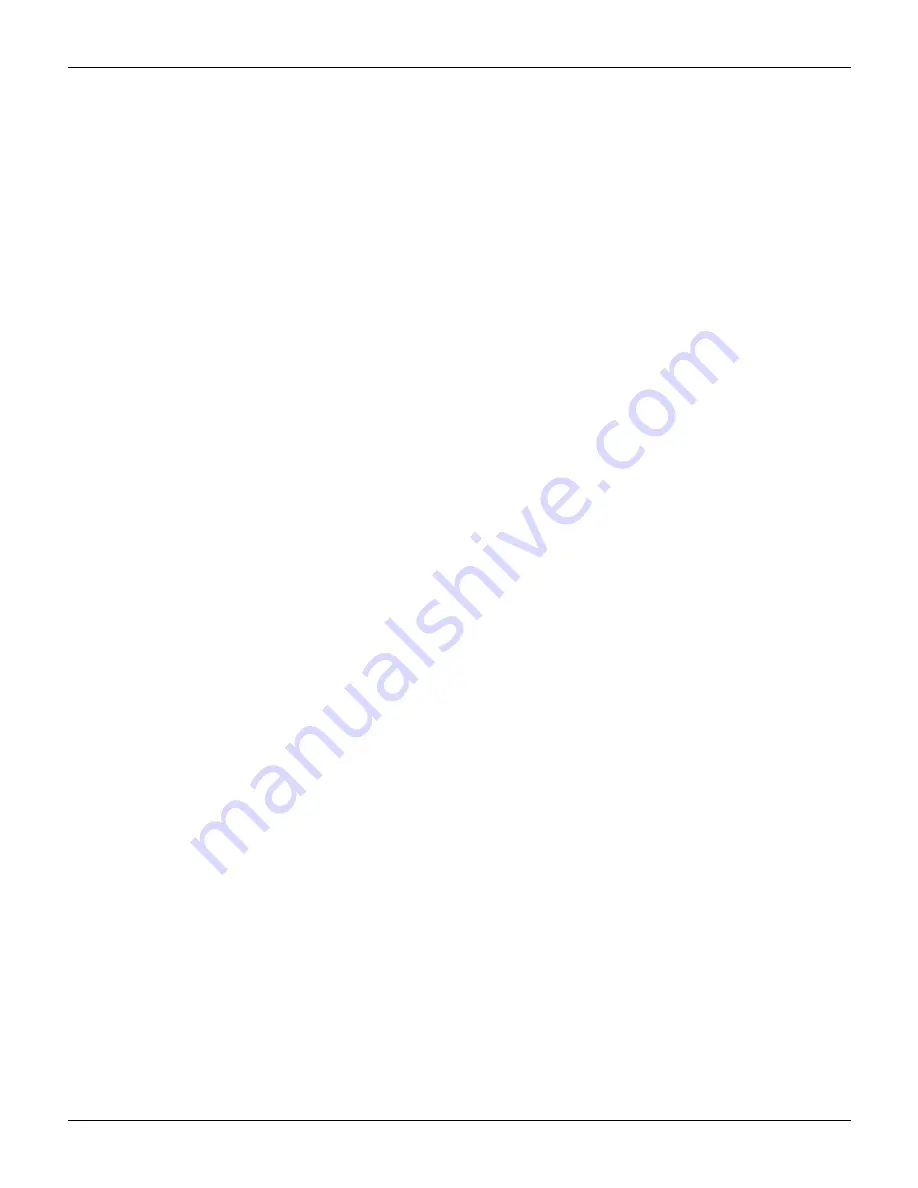
2-48
Return to
2600S-901-01 Rev. C / January 2008
Section 2: TSP Programming
Series 2600 System SourceMeter® Instruments Reference Manual
circle = {clr = "red", diam = 1, setdiam = function(d)
circle["diam"]=d end}
print(circle["clr"])
-- Index using a string; print the clr property.
print(circle["diam"])
-- Index using a string; print the diam property.
circle["setdiam"](2)
-- Change the diam element by calling setdiam method.
print(circle.diam)
-- circle["diam"] is the same as circle.diam; simpler syntax
circle.setdiam(3)
-- Change the diameter of the circle again.
print(circle.diam)
-- Print diam property again using simple syntax.
Output of code above:
red
1
2
3
Precedence
Operator precedence in TSL follows the table below, from higher to lower priority:
^
not
- (unary)
*
/
+
-
.. (concatenation)
<
>
<=
>=
~=
==
and
or
All operators are left associative, except for ‘
^
’ (exponentiation) and ‘
..
’, which are right
associative. Therefore, the following expressions on the left are equivalent to those on the right:
a+i < b/2+1
(a+i) < ((b/2)+1)
5+x^2*8
5+((x^2)*8)
a < y and y <= z
(a < y) and (y <= z)
-x^2
-(x^2)
x^y^z x^(y^z)
Logical operators
The logical operators are
and
,
or
, and
not
. Like control structures, all logical operators consider
false
and
nil
as false and anything else as true.
The operator
and
returns its first argument if it is false, otherwise it returns its second argument.
The operator
or
returns its first argument if it is not false; otherwise it returns its second argument:
print(4 and 5)