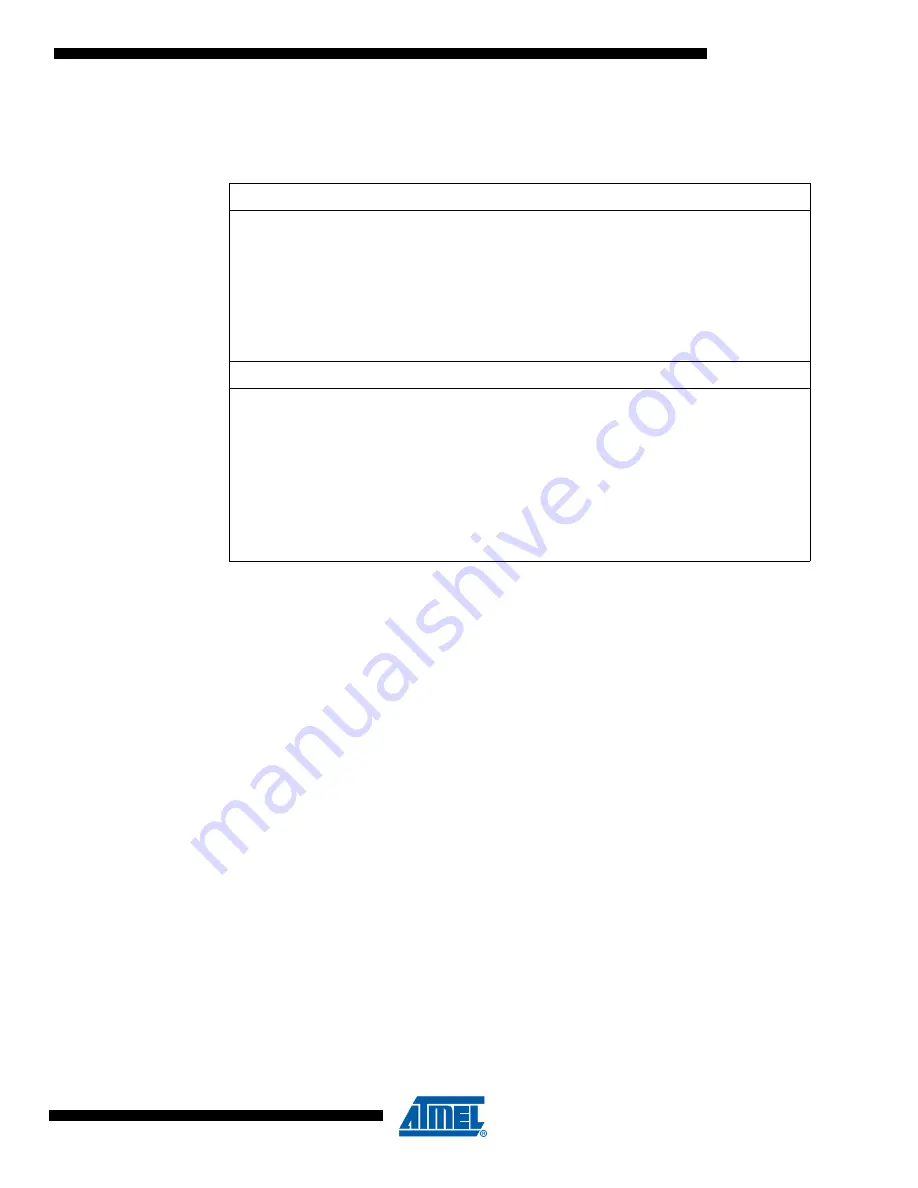
181
2467S–AVR–07/09
ATmega128
The following code example shows a simple USART receive function based on polling of the
Receive Complete (RXC) flag. When using frames with less than eight bits the most significant
bits of the data read from the UDR will be masked to zero. The USART has to be initialized
before the function can be used.
Note:
1. See “About Code Examples” on page 9.
The function simply waits for data to be present in the receive buffer by checking the RXC flag,
before reading the buffer and returning the value.
Assembly Code Example
USART_Receive:
;
Wait for data to be received
sbis
UCSRA, RXC
rjmp
USART_Receive
;
Get and return received data from buffer
in
r16, UDR
ret
C Code Example
unsigned char
USART_Receive(
void
)
{
/*
Wait for data to be received
*/
while
( !(UCSRA & (1<<RXC)) )
;
/*
Get and return received data from buffer
*/
return
UDR;
}