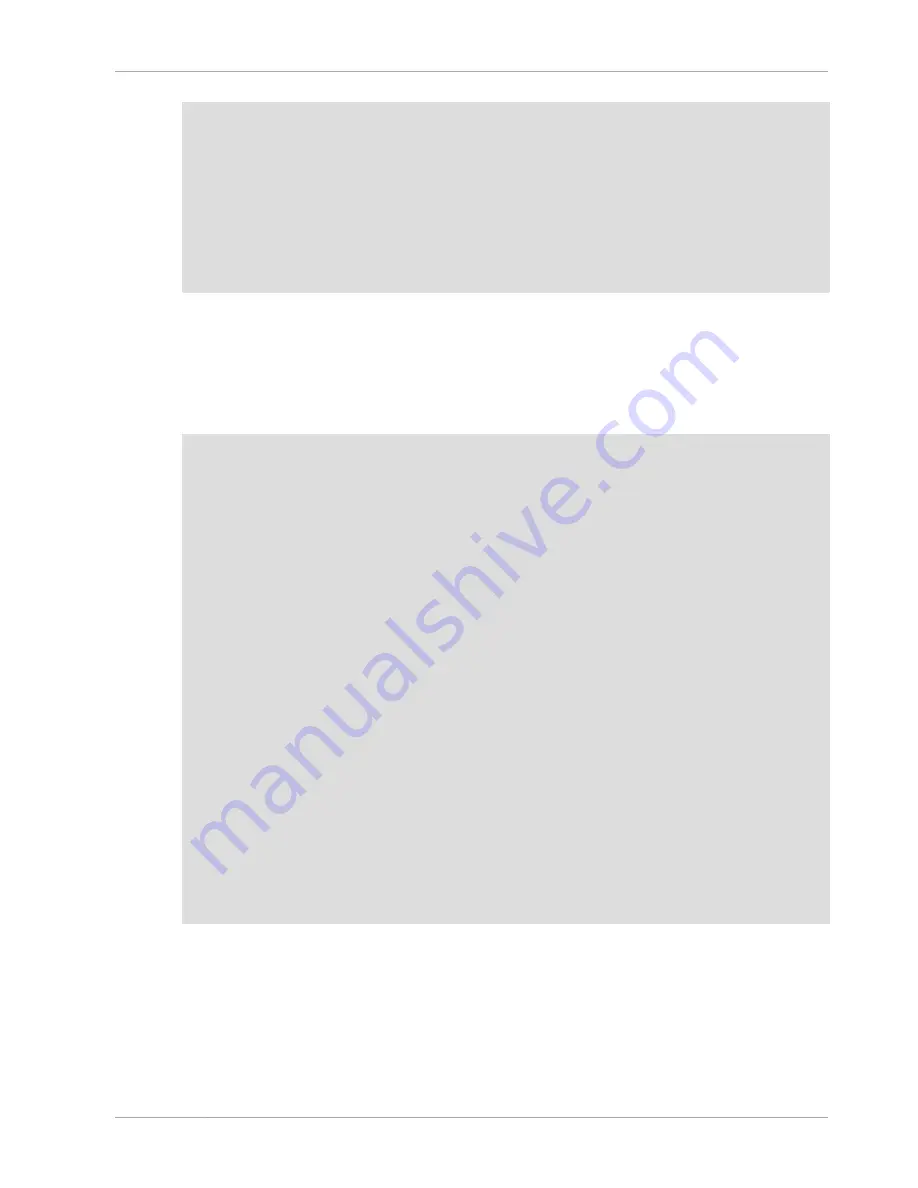
Connector/Net Tutorials
1859
string sql = "INSERT INTO Country (Name, HeadOfState, Continent) VALUES ('Disneyland','Mickey Mouse', 'North America')";
MySqlCommand cmd = new MySqlCommand(sql, conn);
cmd.ExecuteNonQuery();
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
}
conn.Close();
Console.WriteLine("Done.");
}
}
The query is constructed, the command object created and the
ExecuteNonQuery
method called on
the command object. You can access your MySQL database with the
mysql
command interpreter and
verify that the update was carried out correctly.
Finally, you will see how the
ExecuteScalar
method can be used to return a single value. Again, this
is straightforward, as a
MySqlDataReader
object is not required to store results, a simple variable will
do. The following code illustrates how to use
ExecuteScalar
:
using System;
using System.Data;
using MySql.Data;
using MySql.Data.MySqlClient;
public class Tutorial4
{
public static void Main()
{
string connStr = "server=localhost;user=root;database=world;port=3306;password=******;";
MySqlConnection conn = new MySqlConnection(connStr);
try
{
Console.WriteLine("Connecting to MySQL...");
conn.Open();
string sql = "SELECT COUNT(*) FROM Country";
MySqlCommand cmd = new MySqlCommand(sql, conn);
object result = cmd.ExecuteScalar();
if (result != null)
{
int r = Convert.ToInt32(result);
Console.WriteLine("Number of countries in the World database is: " + r);
}
}
catch (Exception ex)
{
Console.WriteLine(ex.ToString());
}
conn.Close();
Console.WriteLine("Done.");
}
}
This example uses a simple query to count the rows in the
Country
table. The result is obtained by
calling
ExecuteScalar
on the command object.
20.2.4.1.3. Working with Decoupled Data
Previously, when using
MySqlDataReader
, the connection to the database was continually
maintained, unless explicitly closed. It is also possible to work in a manner where a connection is only
established when needed. For example, in this mode, a connection could be established to read a
chunk of data, the data could then be modified by the application as required. A connection could then
Summary of Contents for 5.0
Page 1: ...MySQL 5 0 Reference Manual ...
Page 18: ...xviii ...
Page 60: ...40 ...
Page 396: ...376 ...
Page 578: ...558 ...
Page 636: ...616 ...
Page 844: ...824 ...
Page 1234: ...1214 ...
Page 1427: ...MySQL Proxy Scripting 1407 ...
Page 1734: ...1714 ...
Page 1752: ...1732 ...
Page 1783: ...Configuring Connector ODBC 1763 ...
Page 1793: ...Connector ODBC Examples 1773 ...
Page 1839: ...Connector Net Installation 1819 2 You must choose the type of installation to perform ...
Page 2850: ...2830 ...
Page 2854: ...2834 ...
Page 2928: ...2908 ...
Page 3000: ...2980 ...
Page 3122: ...3102 ...
Page 3126: ...3106 ...
Page 3174: ...3154 ...
Page 3232: ...3212 ...