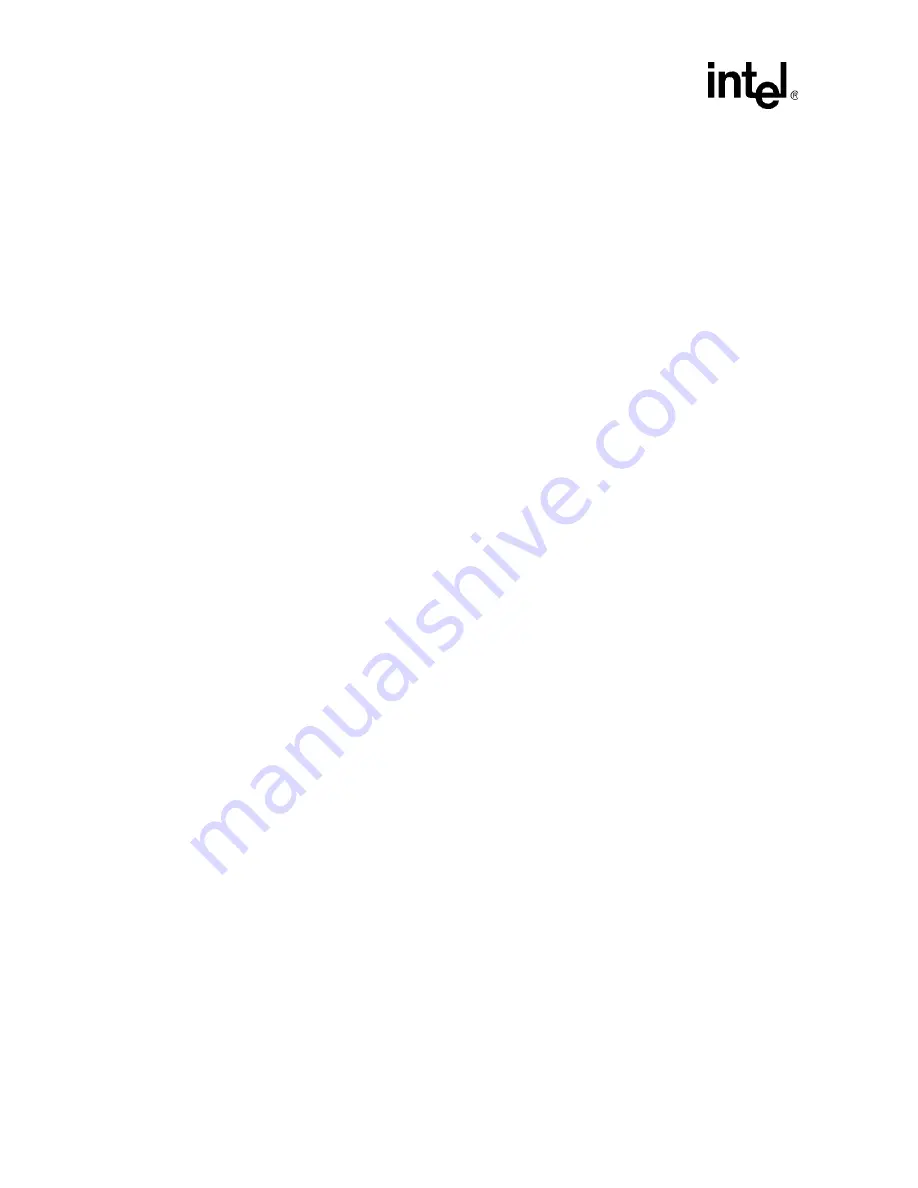
204
January, 2004
Developer’s Manual
Intel XScale® Core
Developer’s Manual
Optimization Guide
A.4.4.10.
Pointer Prefetch
Not all looping constructs contain induction variables. However, prefetching techniques can still be
applied. Consider the following linked list traversal example:
while(p) {
do_something(p->data);
p = p->next;
}
The pointer variable p becomes a pseudo induction variable and the data pointed to by p->next can
be prefetched to reduce data transfer latency for the next iteration of the loop. Linked lists should
be converted to arrays as much as possible.
while(p) {
prefetch(p->next);
do_something(p->data);
p = p->next;
}
Recursive data structure traversal is another construct where prefetching can be applied. This is
similar to linked list traversal. Consider the following pre-order traversal of a binary tree:
preorder(treeNode *t) {
if(t) {
process(t->data);
preorder(t->left);
preorder(t->right);
}
}
The pointer variable t becomes the pseudo induction variable in a recursive loop. The data
structures pointed to by the values t->left and t->right can be prefetched for the next iteration of
the loop.
preorder(treeNode *t) {
if(t) {
prefetch(t->right);
prefetch(t->left);
process(t->data);
preorder(t->left);
preorder(t->right);
}
}
Note the order reversal of the prefetches in relationship to the usage. If there is a cache conflict and
data is evicted from the cache then only the data from the first prefetch is lost.