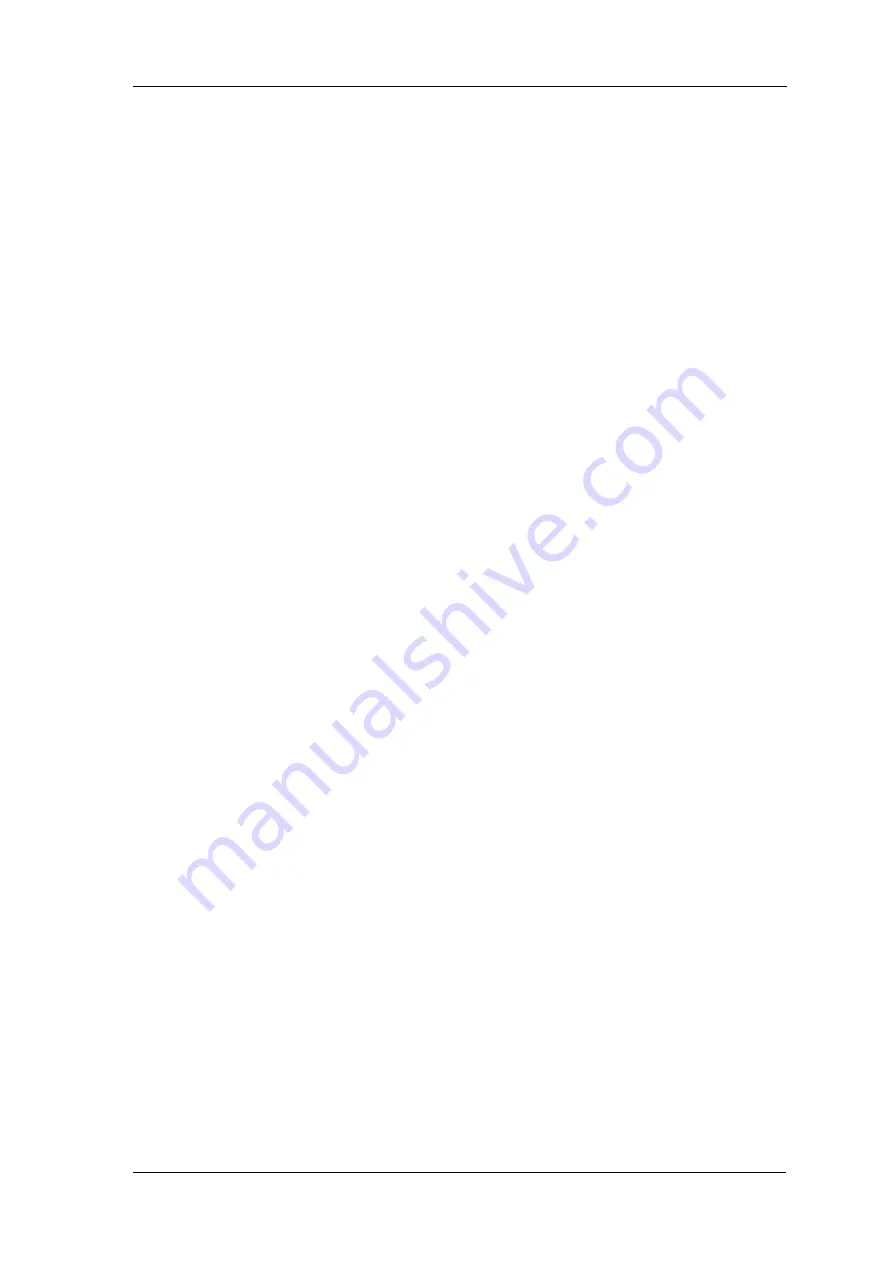
SIGLENT
134 SSG5000X Programming Guide
{
/* This code demonstrates sending synchronous read & write commands */
/* to an USB Test & Measurement Class (USBTMC) instrument using */
/* NI-VISA */
/* The example writes the "*IDN?\n" string to all the USBTMC */
/* devices connected to the system and attempts to read back */
/* results using the write and read functions. */
/* The general flow of the code is */
/* Open Resource Manager */
/* Open VISA Session to an Instrument */
/* Write the Identification Query Using viPrintf */
/* Try to Read a Response With viScanf */
/* Close the VISA Session */
/***********************************************************/
ViSession defaultRM;
ViSession instr;
ViUInt32 numInstrs;
ViFindList findList;
ViStatus status;
char
instrResourceString[VI_FIND_BUFLEN];
unsigned
char
buffer[100];
int
i;
/** First we must call viOpenDefaultRM to get the manager
* handle. We will store this handle in defaultRM.*/
status = viOpenDefaultRM (&defaultRM);
if
(status<VI_SUCCESS)
{
printf ("Could not open a session to the VISA Resource Manager!\n");
return
status;
}
/* Find all the USB TMC VISA resources in our system and store the number of resources in the system
in numInstrs.*/
status = viFindRsrc (defaultRM, "USB?*INSTR", &findList, &numInstrs, instrResourceString);
if
(status<VI_SUCCESS)
{
printf ("An error occurred while finding resources.\nPress 'Enter' to continue.");
fflush(stdin);
getchar();
viClose (defaultRM);
return
status;
}
/** Now we will open VISA sessions to all USB TMC instruments.
* We must use the handle from viOpenDefaultRM and we must