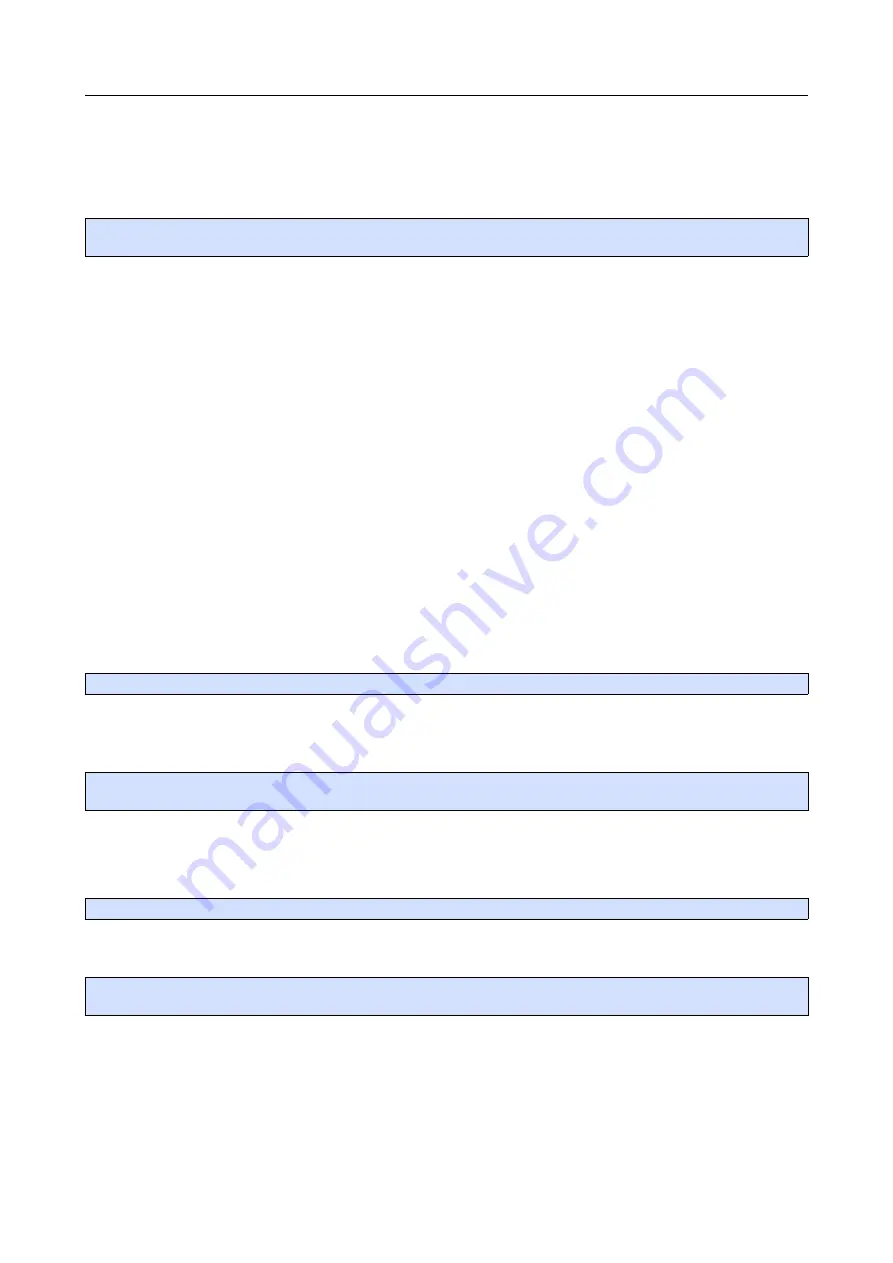
RP6 ROBOT SYSTEM - 4. Programming the RP6
For example if you would like to read register 22 from a slave device with address 10:
I2CTWI_transmitByte(10, 22);
uint8_t result = I2CTWI_readByte(10);
The following function allows you to read several bytes:
void I2CTWI_readBytes(uint8_t targetAdr, uint8_t * messageBuffer,
uint8_t numberOfBytes);
Example:
I2CTWI_transmitByte(10, 22);
uint8_t results[6];
I2CTWI_readBytes(10,results,5);
This code snippet reads 5 bytes from register 22 of a slave device with address 10. If
this data is really read from Register 22 varies from slave to slave device. Some will
increment the register number automatically (just like the slave code of the RP6Lib-
rary does) and other work completely different. You have to check the documentation
of your devices about this!
Reading data in background is a bit more complex task. First of all you will have to
start a request for a number of bytes from a slave. A background process will be star-
ted to retrieve these bytes from the slave device. In the meantime the controller is al-
lowed to perform other jobs, intermittently being disturbed by the interrupt routine.
Of course, we will frequently have to call a function from the main loop to check for
the arrival of requested data from a slave device or for a failure condition in the com-
munication process. On data arrival, this function automatically calls a predefined
Event Handler function for further data processing and it may immediately start re-
trieving the next set of data from other registers. Each request will be managed by its
own ID.
void task_I2CTWI(void)
is the function, which has to be called frequently from the main loop. This task has
been designed to check all transfers for error-free completion and to call the Event
Handler as required.
void I2CTWI_requestDataFromDevice(uint8_t requestAdr, uint8_t requestID,
uint8_t numberOfBytes)
This function allows you to request data from a slave device. After calling the function
a background process will automatically retrieve the data as described above.
Subsequently we can fetch the data by calling the function:
void I2CTWI_getReceivedData(uint8_t *msg, uint8_t msgSize)
We can fetch the data as soon as the Event Handler is called. The Handler has to be
registered by calling the function:
void I2CTWI_setRequestedDataReadyHandler(void (*requestedDataReadyHandler)
(uint8_t))
The Event Handler must have the following signature pattern:
void I2C_requestedDataReady(uint8_t dataRequestID)
This Handler will be called with the ID of the data request as parameter. We can use
the ID to differentiate between different slave devices.
- 109 -