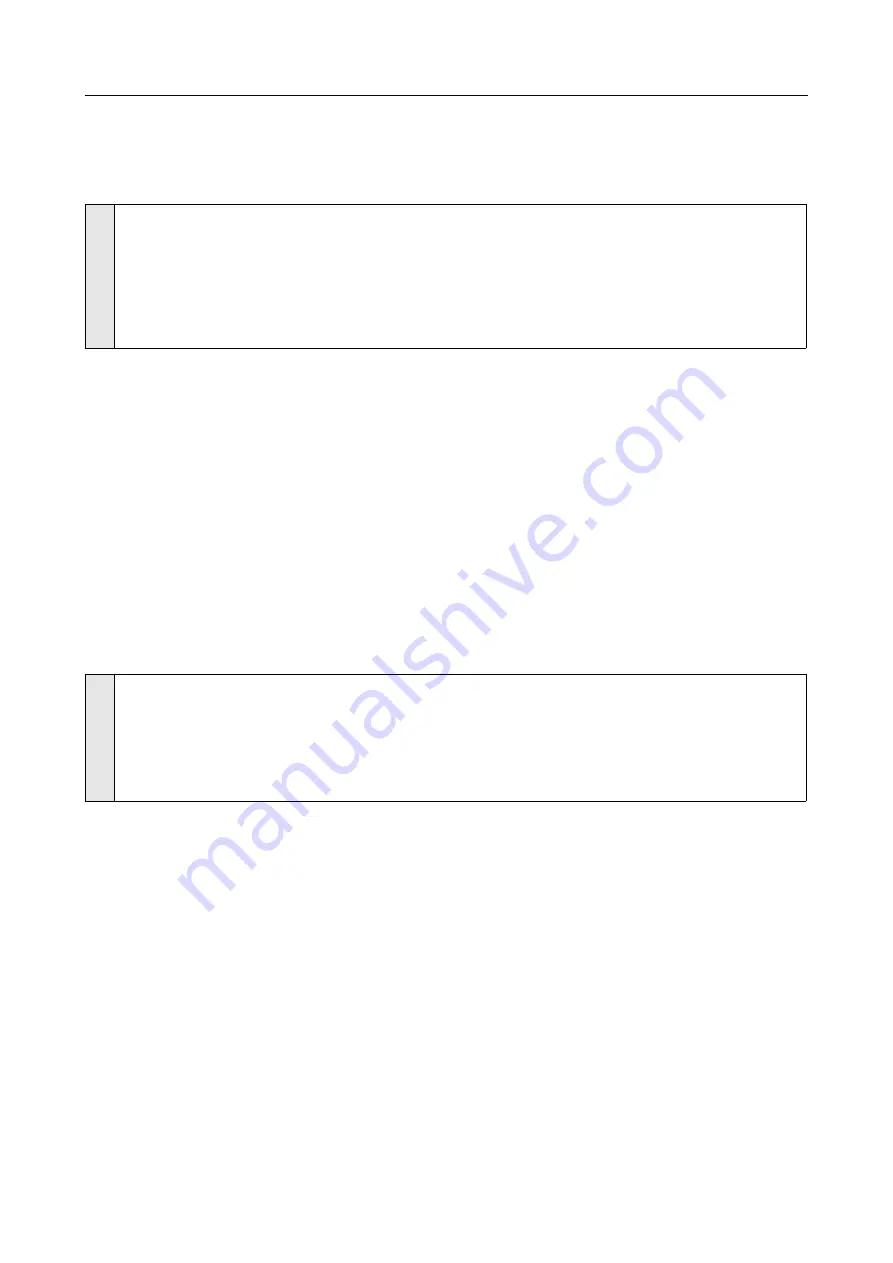
RP6 ROBOT SYSTEM - 4. Programming the RP6
4.4.7. Loops
We need loops if operations need to be repeated a number of times.
Let's demonstrate the basic principle in an example:
1
2
3
4
5
6
7
8
uint8_t
i =
0
;
while
(
i
<
10
)
// as long as i is less than 10...
{
// ... repeat the following code:
writeString
(
"i="
);
// output "i=",
writeInteger
(
i
,
DEC
);
// output the "DECimal" value of i and ...
writeChar
(
'\n'
);
// ... a line-break.
i++
;
// increment i.
}
Obviously the code snippet contains a “while”-conditional loop, generating the se-
quence: “i=0\n”, “i=1\n”, “i=2\n”, ... “i=9\n”. Following the while-conditional header
“while(i < 10)” the block surrounded by the accolades will be repeated as long as the
condition is true. In plain English this may be read as: “Repeat the following block as
long as i is less than 10”. As we have an initial value of i = 0 and increment i at every
loop-cycle, the program will be executing the loop-body 10 times and output the num-
bers from 0 to 9. In the loop-header, you can use the same conditions as in if-condi-
tions.
Beneath the while-loop we can use the “for”-loop which provides similar functionality,
but offers extended features for the loop-header definition.
A sample code snippet may illustrate the for-loop:
1
2
3
4
5
6
7
uint8_t
i;
// we will not initialize i here, but in the loop-header!
for
(
i
=
0
;
i
<
10
;
i++
)
{
writeString
(
"i="
);
writeInteger
(
i
,
DEC
);
writeChar
(
'\n'
);
}
This for-loop will generate output identical to the previous while-loop. However, we
could implement several things within the loop-header.
Basically the for-loop is structured as follows:
for ( <initialize control variable> ; <terminating condition> ; <modify the control variable> )
{
<command block>
}
Working with microcontrollers, you will often need infinite loops, which virtually may
be repeated eternally. In fact, most microcontroller programs contain at least one in-
finite loop – either to put the program into a well know state for terminating the regu-
lar program flow, or by endlessly performing operations until the device is switched
off.
- 71 -