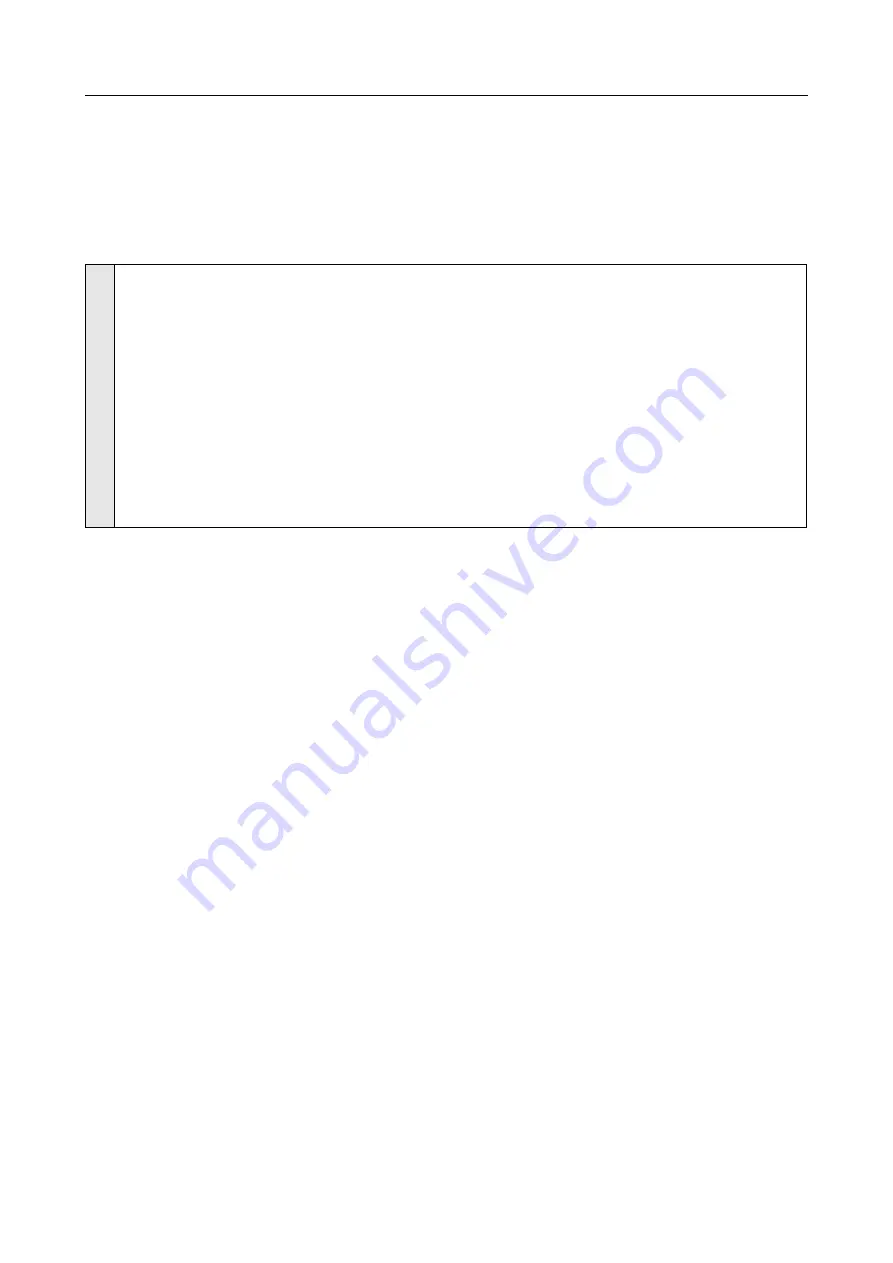
RP6 ROBOT SYSTEM - 4. Programming the RP6
4.4.6. Switch-Case
Often we will have to compare a variable to a great number of different values and
decide to execute further program code according to the result of these comparisons.
Of course, we could use a great number of if-then-else conditional statements, but the
language provides a more elegant method by using a switch-case-construct.
A small example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
uint8_t
x
=
3
;
switch
(
x
)
{
case
1
:
writeString
(
"x=1\n"
);
break
;
case
2
:
writeString
(
"x=2\n"
);
break
;
case
3
:
writeString
(
"x=3\n"
);
// At this point, "break" is missing,
case
4
:
writeString
(
"Hello\n"
);
// causing the program to proceed
case
5
:
writeString
(
"over\n"
);
// with the next two lines
case
6
:
writeString
(
"there!\n"
);
break
;
// and stop here!
case
44
:
writeString
(
"x=44\n"
);
break
;
// The program will jump to this line if none of the previous
// conditions is met:
default
:
writeString
(
"x is something else!\n"
);
break
;
}
This code snippet works quite similar compared to the previous example with an “if-
else-if-else-if-else...”-conditional structure, but now we use case-branches instead.
There is one main difference – if one condition is true, all the following case-branches
will be executed. If you do not want that – just add a “break” instruction and it will
quit the switch-case construct there.
The output of the example above would be (for the default value x = 3):
x=3
Hello
over
there!
Setting x = 1 would result in an output of “
x=1\n”
and x = 5 would result in an output
of:
over
there!
You may now understand the “break”-instruction will terminate the case-branches. If
you omit the “break”-instruction, the program will be wading through any following in-
structions until either the end of the switch-construct or another “break” is reached .
If we preset the value x = 7, none of the branches will be true. The program now ex-
ecutes the “default”-branch, resulting in an output of :
"The value of x is something
else!\n".
Of course the text output is only an example, but real programs may be using these
constructs to generate various different movements with the robot. Several example
programs use switch-case constructs for finite state machines to implement a simple
behaviour based robot.
- 70 -