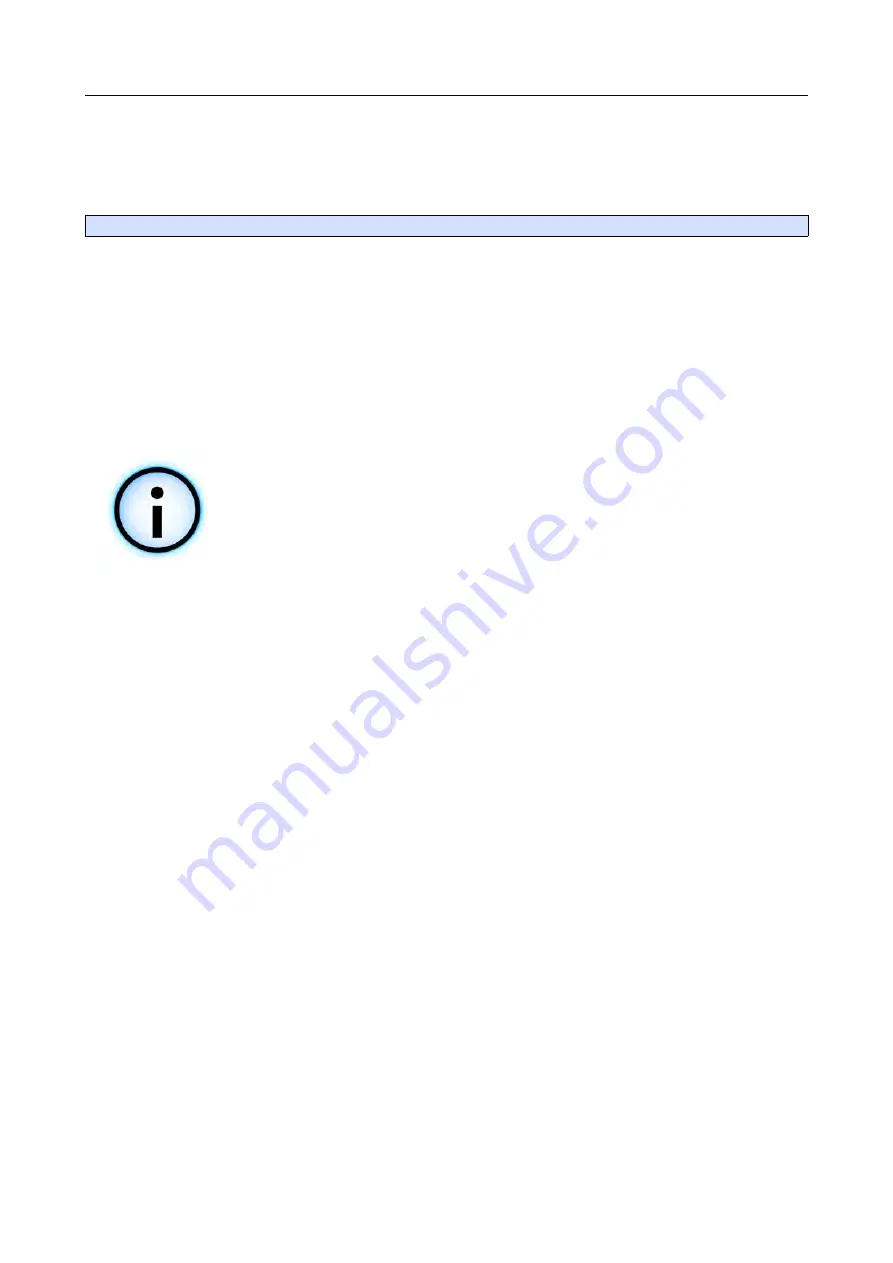
RP6 ROBOT SYSTEM - 4. Programming the RP6
4.6.5. Read ADC values (Battery, Motorcurrent and Light sensors)
There are a lot of sensors connected to the ADC (Analog to Digital Converter), as de-
scribed in chapter 2. Of course, the RP6Library provides a function to read the meas-
ured ADC values:
uint16_t readADC(uint8_t channel)
This function returns a 10 Bit value (0...1023) and requires a 16 Bit variable for
sensor values.
The following channels can be read:
ADC_BAT
--> Battery voltage sensor
ADC_MCURRENT_R
--> Motorcurrent sensor for the right motor
ADC_MCURRENT_L
--> Motorcurrent sensor for the left motor
ADC_LS_L
--> Left light sensor
ADC_LS_R
--> Right light sensor
ADC_ADC0
--> Free ADC channel for your own sensor devices
ADC_ADC1
--> Free ADC channel for your own sensor devices
Hint: the two connectors for the free ADC channels are not popu-
lated. You may solder connectors with standard 2.54mm grid and
maybe additionally insert two 100nF capacitors and a large 470µF
Elco, just in case your sensor circuitry required high peak current,
like Sharp IR-distance-sensor do...
This requires some soldering-experience! If you are unexperienced,
it may be a better idea to go for an extension module!
Examples:
uint16_t ubat = readADC(ADC_BAT);
uint16_t iMotorR = readADC(ADC_MCURRENT_R);
uint16_t iMotorL = readADC(ADC_MCURRENT_L);
uint16_t lsL = readADC(ADC_LS_L);
uint16_t lsR = readADC(ADC_LS_R);
uint16_t free_adc0 = readADC(ADC_ADC0);
uint16_t free_adc1 = readADC(ADC_ADC1);
if(ubat < 580) writeString_P("Warning! Low battery level!");
Basically the 5V supply is used as reference voltage, but the function could be modi-
fied such that the internal ATMEGA32's 2.56V reference voltage is used instead (see
the MEGA32 data sheet). The standard RP6 sensors do not require this usually.
It makes sense to perform several ADC measurements subsequently, to store the res-
ults in an array and to calculate the average and/or Minimum/Maximum value before
processing the ADC output any further.
Processing several values can reduce measurement errors. As an example where “av-
eraging” methods are required, we may consider the battery voltage measurement.
The Battery voltage will vary a lot under heavy load, especially with alternating load
conditions like caused by the motors.
- 91 -