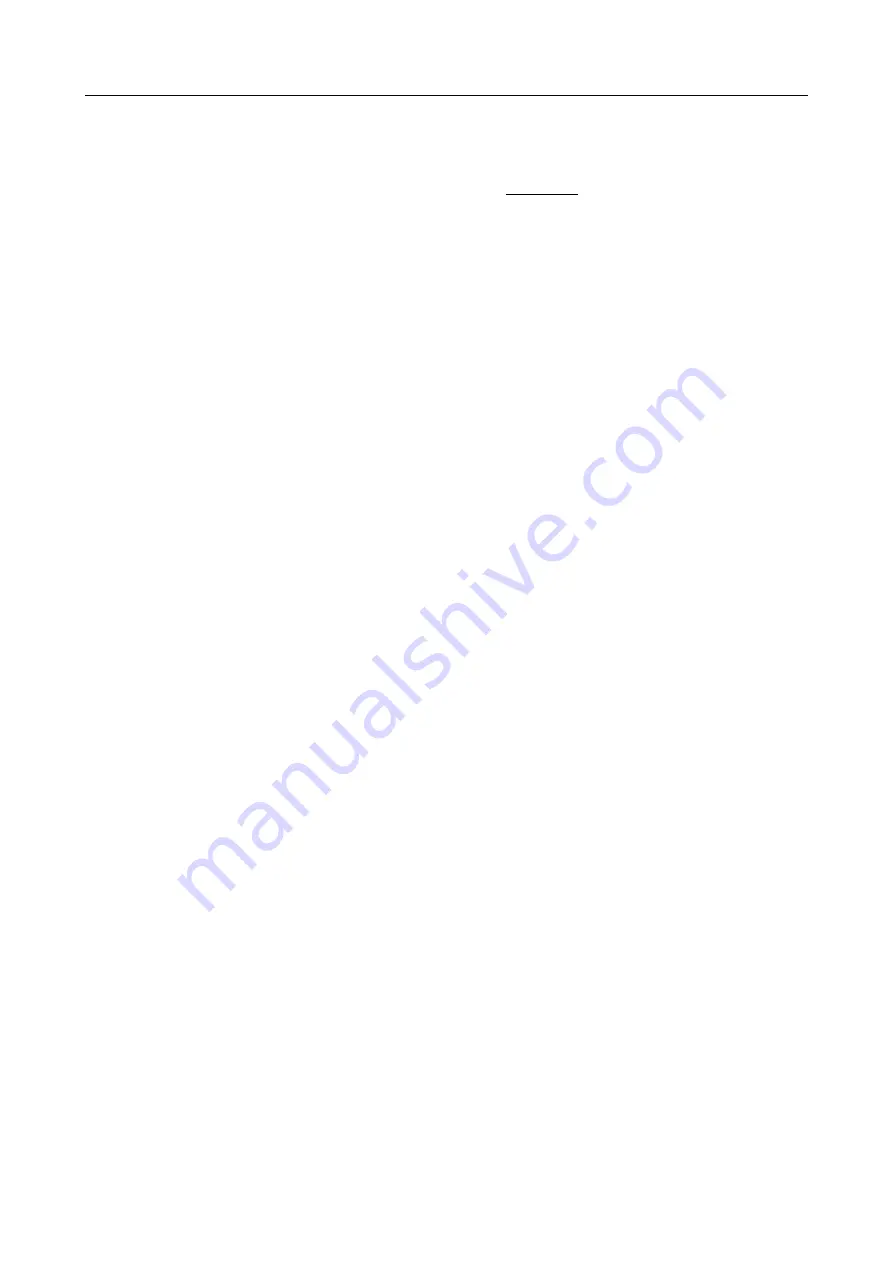
RP6 ROBOT SYSTEM - 4. Programming the RP6
In order to use a variable in a program we have to declare it first by defining the data
type, a name and eventually an initial value for this variable. The name must start
with an alphabetic character (including the underscore “_”), and may contain num-
bers. However the variable's naming convention
excludes
a great number of special
characters, e.g. “äöüß#'[]²³|*+-.,<>%&/(){}$§=´°?!^”.
Variable names are case sensitive, which implies aBc and abC are different variables!
Traditionally, programmers use lower case characters at least for the leading character
of variable names.
The following keywords are already reserved and are NOT useable as variable names,
function names or any other symbols:
auto
default
float
long
sizeof
union
break
do
for
register static
unsigned
case
double
goto
return
struct
void
char
else
if
short
switch
volatile
const
enum
int
signed
typedef
while
continue extern
Furthermore the types float and double are used for floating point numbers, but we
prefer to avoid usage of these data types on small AVR microcontroller. Floating point
numbers are very computation time and memory intensive and usually we are able to
work perfectly well with integers. Most RP6 programs will not require floating num-
bers.
Declaring variables is extremely simple, which may be demonstrated by declaring a
variable named x:
char x;
After its declaration the variable x is valid in the following program lines and may be
used e.g. by assigning a value of 10 to it:
x = 10;
Alternatively we may assign a value to another variable y directly at declaration:
char y = 53;
Basic arithmetic operations may be used as usual:
signed char z; // please note the “signed” in front of char!
z = x + y; // z gets the value z = x + y = 10 + 53 = 63
z = x – y; // z gets the value z = 10 – 53 = -43
z = 10 + 1 + 2 – 5; // z = 8
z = 2 * x; // z = 2 * 10 = 20
z = x / 2; // z = 10 / 2 = 5
The programming language also provides some useful abbreviations:
z += 10; // corresponds to: z = z + 10; this means z = 15 in this case
z *= 2; // z = z * 2 = 30
z -= 6; // z = z - 6 = 24
z /= 4; // z = z / 4 = 8
- 67 -