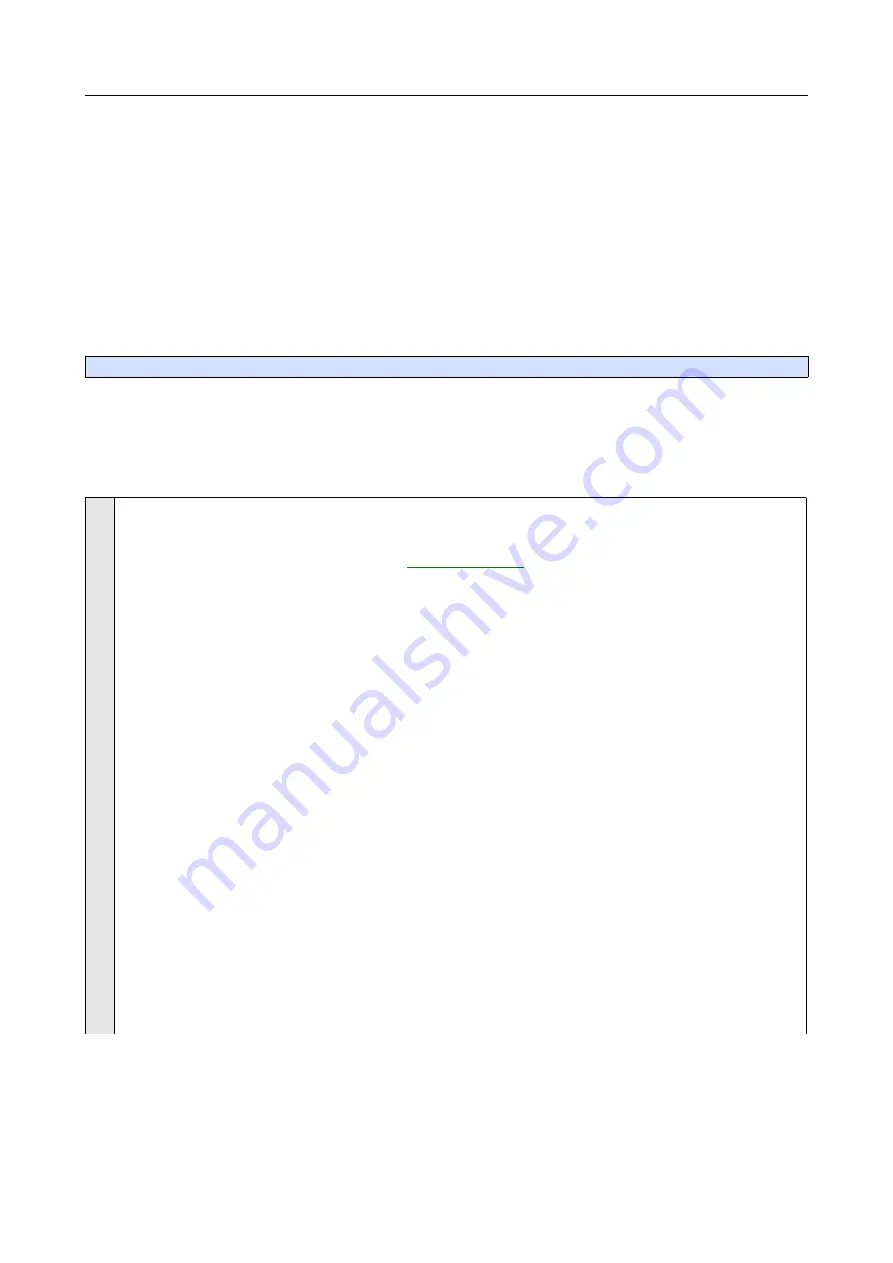
RP6 ROBOT SYSTEM - 4. Programming the RP6
C allows us to define pointers to functions and call these functions without pre-defin-
ing the function in the library. Usually a function needs to be defined in our Library at
the time of compilation in order to be callable.
This method allows us to use self-defined functions as so-called “Event Handlers”.
Pressing down a bumper will automatically result in calling a predefined dedicated
function (within 50ms). This special function must be registered as an Event Handler
and will have to provide a specific signature: the function must not return a value and
has no parameter (both return value and parameter must be “void”). Therefore the
function's signature will have to look like:
void bumpersStateChanged(void)
. For
example you may register the Event Handler at the very beginning of the main
function. Registering the Event Handler can be done with the following function:
void BUMPERS_setStateChangedHandler(void (*bumperHandler)(void))
You do not have to exactly understand this command – to make a long story short this
function expects a pointer to a function as parameter...
We will explain this in a simple example:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
#include "RP6RobotBaseLib.h"
// Our
"
Event Handler
"
function for the bumpers.
// This function will be called
automatically
by the RP6Library:
void
bumpersStateChanged
(
void
)
{
writeString_P
(
"\nBumper status changed:\n"
);
if
(
bumper_left
)
writeString_P
(
" - Left bumper pressed down!\n"
);
else
writeString_P
(
" - Left bumper released!\n"
);
if
(
bumper_right
)
writeString_P
(
" - Right bumper pressed down!\n"
);
else
writeString_P
(
" - Right bumper released\n"
);
}
int
main
(
void
)
{
initRobotBase
();
// Register the Event Handler:
BUMPERS_setStateChangedHandler
(
bumpersStateChanged
);
while
(
true
)
{
task_Bumpers
();
// Automatically check bumpers at 50ms intervals
}
return
0
;
}
- 89 -