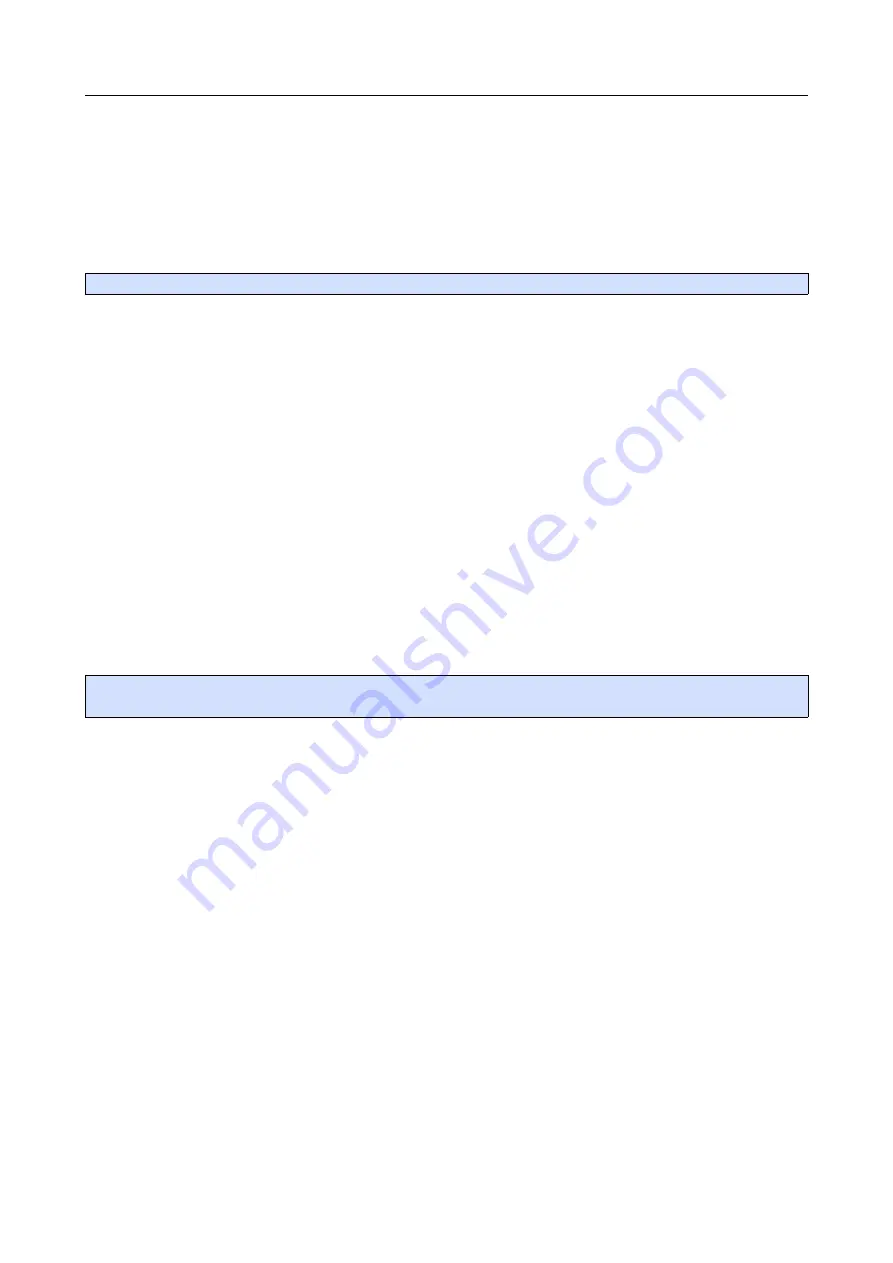
RP6 ROBOT SYSTEM - 4. Programming the RP6
4.6.2. UART Functions (serial interface)
A few of the RP6Library's functions have been used in the previous C crash course
already, such as the UART functions. These functions allow us to transfer text mes-
sages through the robot's serial interface to and from the PC (or to another microcon-
troller).
4.6.2.1. Transmitting data
void writeChar(char ch)
This function transmits a single 8-Bit ASCII character via the serial interface.
Usage is simple:
writeChar('A');
writeChar('B');
writeChar('C');
This would output “ABC”. The function can also transfer ASCII codes directly, e.g.:
writeChar(65);
writeChar(66);
writeChar(67);
This would also result in an output of “ABC”, because any ASCII character may be rep-
resented by a number. The number 65 refers to the character 'A'. A special communic-
ation software can also directly interpret the binary values if necessary.
You will frequently need something like:
writeChar('\n');
to start a new line in the terminal software.
void writeString(char *string)
and
writeString_P(STRING)
These functions are important for debugging programs, as they allow transmitting any
text messages to the PC. Of course they may be useful for data transfers as well.
We will now have to explain the difference between writeString and writeString
_P
.
Working with writeString_P will cause the text strings to be stored in Flash-ROM (
P
ro-
gram Memory) only and of course we will have to read these strings back from Flash-
ROM for output. In contrast, for writeString the strings will get stored into RAM
and
the Flash-ROM, which requires a double amount of memory. Please remember the rel-
atively small 2KB RAM! So, if you have to output fixed text strings you should prefer
using writeString_P. Of course for transferring dynamic data, which has to be available
in RAM anyway, writeString
must
be used.
Using the corresponding function is just as easy as using writeChar (please note the
double quotes instead of the apostrophe used for writeChar...):
writeString("ABCDEFG");
which will output “ABCDEFG”, but as mentioned above, this string will get stored in
ROM and will be loaded into RAM at startup.
writeString_P("ABCDEFG");
will equally output “ABCDEFG”, but it does not occupy RAM for the text!
- 80 -