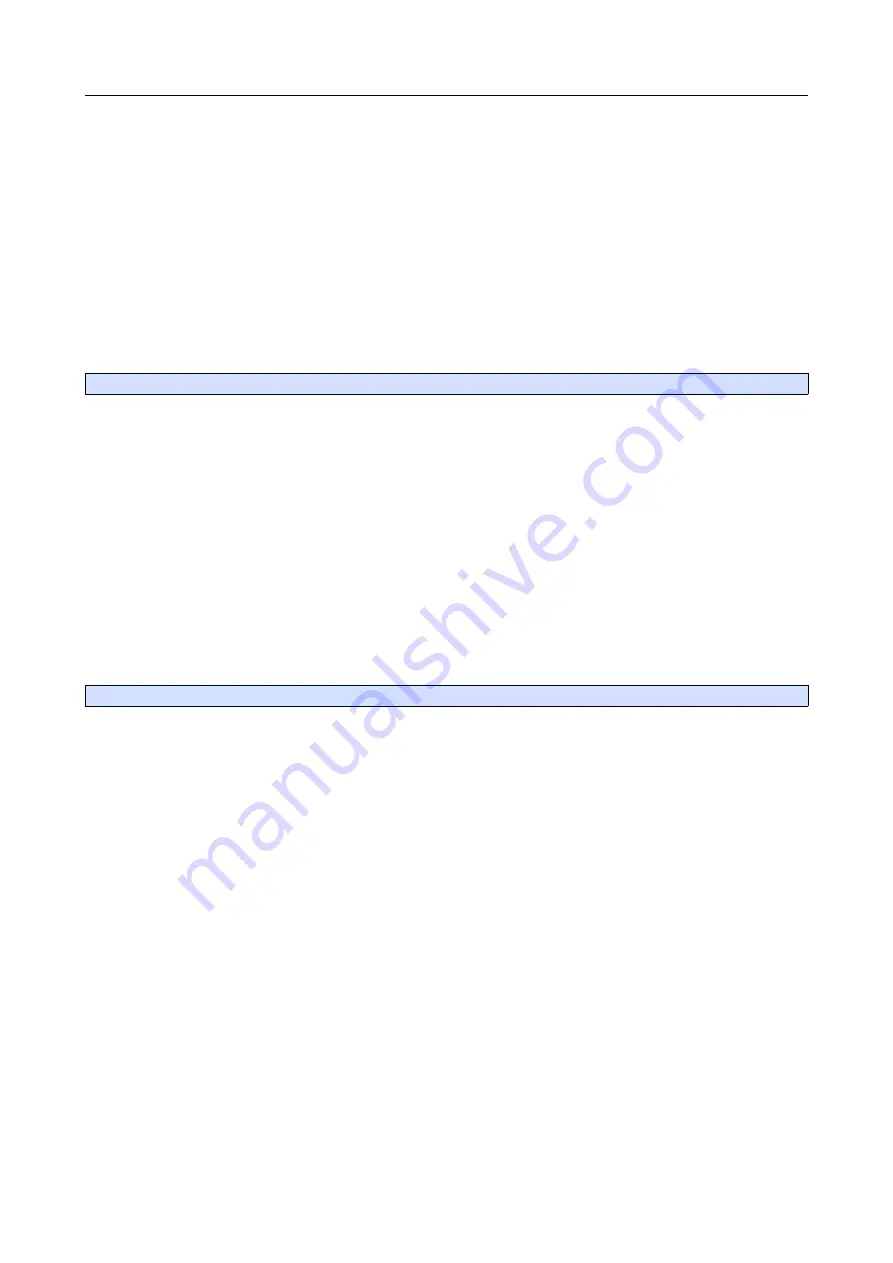
RP6 ROBOT SYSTEM - 4. Programming the RP6
4.6.3. Delay and timer functions
Microcontroller programs often have to be delayed completely for some time, or need
to wait a period of time before a specific action is performed.
The RP6Library also provides functions for these purposes. It uses one of the
MEGA32's timers to achieve relatively accurate delay control, which is independent
from other program flow or interrupts which could disturb delay routines.
You will have to carefully decide where you can use these functions! Using these func-
tions along with automatic speed control and ACS (will be explained later) may cause
problems! If you need to use automatic speed control or ACS, please use very short
delays of less than 10 milliseconds only! Instead of blocking delays, you may prefer
the “stopwatch” functions instead, which will be discussed in the following section.
void sleep(uint8_t time)
This function will stop normal program execution for a predefined period of time. The
delay is specified with a resolution of 100µs (100µs = 0.1ms = 0.0001s, which is ex-
tremely short for human perception...). The use of an 8 bit sized variable allows us to
define delays up to 25500µs = 25.5ms. While the normal program is “sleeping”, inter-
rupts will still be processed immediately. This will only delay the normal program's ex-
ecution. As mentioned before, it uses a hardware timer and is not influenced too bad
by interrupt events.
Examples:
sleep(1); // 100µs delay
sleep(10); // 1ms delay
sleep(100); // 10ms delay
sleep(255); // 25.5ms delay
void mSleep(uint16_t time)
Whenever you need long delays, you may prefer mSleep, which allows to specify
delay period in milliseconds. The maximum delay period is 65535ms, or 65.5 seconds.
Examples:
mSleep(1); // 1ms delay
mSleep(100); // 100ms delay
mSleep(1000); // 1000ms = 1s delay
mSleep(10000); // 10 seconds delay
mSleep(65535); // 65.5 seconds delay
Stopwatches
The problem with these standard delay functions is, that they will stop the normal
program flow completely. This may be unacceptable, if only a specific part of the pro-
gram needs to wait for a period of time, whereas other parts are supposed to continue
with their tasks...
One of the main advantages in using hardware-timers, is independence from the nor-
mal program flow. With these timers, the RP6Library implements universal so-called
“Stopwatches”. The author has chosen this unusual title for similarity with ordinary
Stopwatches. These “Stopwatches” will simplify a great number of jobs. Usually cus-
tomised timer functions for each individual program would be required, but the
- 83 -